As I already mentioned in the comments, if the text you want to shorten is split between the nodes of a subtree, then this gets complicated. It is complicated, because - conceptually, at least - XSLT process the branches of a tree in parallel, and there is no way to pass information from one branch to next, like you can when you're doing a loop.
There are two possible approaches you could take here:
- force the stylesheet to process the nodes sequentially;
- have each text node calculate what has happened in the parallel
branches that precede the current branch in document order.
The second option seems easier, though it is grossly inefficient. Here's a generic example:
XML
In this document, I have inserted a §
as the 100th character of each item's body.
<feed>
<item>
<title>Declaration</title>
<body>
<para>When in the Course of human events, it becomes necessary for one people to <bold>dissolve the political b§ands which have connected them with another</bold>, and to assume among the powers of the earth, the separate and equal station to which the Laws of Nature and of Nature's God entitle them, a decent respect to the opinions of mankind requires that they should declare the causes which impel them to the separation.</para>
<para>We hold these truths to be self-evident, that all men are created equal, that they are endowed by their Creator with certain unalienable Rights, that among these are Life, Liberty and the pursuit of Happiness.</para>
</body>
</item>
<item>
<title>Lorem Isum</title>
<body>
<para>Lorem ipsum dolor sit amet consectetuer adipiscing elit. <bold>Nam interdum ante quis <italic>erat pellentesque e§lementum.</italic> Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus.</bold> Ut molestie quam sit amet ligula.</para>
<para>In enim. Duis dapibus hendrerit quam. Donec hendrerit lectus vel nunc. Vestibulum sit amet pede nec neque dignissim vehicula.</para>
<para>Class aptent taciti sociosqu ad litora torquent per conubia nostra, per inceptos hymenaeos. Phasellus eget ante. Quisque risus leo, dictum sit amet, nonummy sit amet, consectetuer ut, mi.</para>
</body>
</item>
<item>
<title>Subject</title>
<body>
<para>Subject to change without notice.</para>
<para>Not responsible for direct, indirect, incidental or consequential §damages resulting from any defect, error or failure to perform.</para>
<para>May be too intense for some viewers.</para>
</body>
</item>
</feed>
XSLT 2.0
<xsl:stylesheet version="2.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:output method="xml" omit-xml-declaration="yes" version="1.0" encoding="utf-8" indent="yes"/>
<xsl:strip-space elements="*"/>
<xsl:param name="limit" select="100"/>
<xsl:template match="feed">
<body>
<xsl:apply-templates/>
</body>
</xsl:template>
<xsl:template match="item">
<h3><xsl:value-of select="title"/></h3>
<xsl:apply-templates select="body"/>
<hr/>
</xsl:template>
<xsl:template match="body">
<p>
<xsl:apply-templates mode="summary"/>
</p>
</xsl:template>
<xsl:template match="bold" mode="summary">
<b>
<xsl:apply-templates mode="summary"/>
</b>
</xsl:template>
<xsl:template match="italic" mode="summary">
<i>
<xsl:apply-templates mode="summary"/>
</i>
</xsl:template>
<xsl:template match="text()" mode="summary">
<xsl:variable name="text-before">
<xsl:value-of select="ancestor::body//text()[current() >> .]" separator=""/>
</xsl:variable>
<xsl:variable name="used" select="string-length($text-before)" />
<xsl:if test="$used lt $limit">
<xsl:value-of select="substring(., 1, $limit - $used)"/>
<xsl:if test="string-length(.) + $used ge $limit">
<xsl:text>...</xsl:text>
</xsl:if>
</xsl:if>
</xsl:template>
</xsl:stylesheet>
Result
<body>
<h3>Declaration</h3>
<p>When in the Course of human events, it becomes necessary for one people to <b>dissolve the political bß...</b>
</p>
<hr/>
<h3>Lorem Isum</h3>
<p>Lorem ipsum dolor sit amet consectetuer adipiscing elit. <b>Nam interdum ante quis <i>erat pellentesque e§...</i>
</b>
</p>
<hr/>
<h3>Subject</h3>
<p>Subject to change without notice.Not responsible for direct, indirect, incidental or consequential ß...</p>
<hr/>
</body>
Rendered
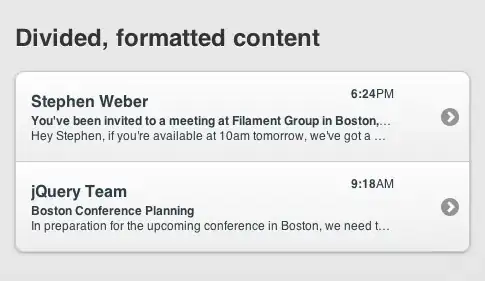
As you can see, each item is cut off after exactly 100 characters, while the internal hierarchy is preserved and each type of node can be processed separately.
Note:
After I wrote this, I went to look at the other question you linked to. The accepted answer there is very similar to this, though I believe mine is a bit simpler and it handles each item separately.