I would use a simple VBA-Collection
and add items with key. The key would be the item itself and because there can't be duplicit keys the collection will contain unique values.
Note: Because adding duplicit key to collection raises error wrap the call to collection-add into a on-error-resume-next.
The function GetUniqueValues
has source-range-values as parameter and retuns VBA-Collection
of unique source-range-values. In the main
method the function is called and the result is printed into Output-Window. HTH.
Sample source range looked like this:
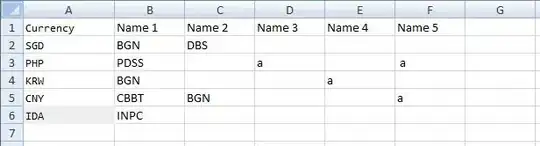
Option Explicit
Sub main()
Dim uniques As Collection
Dim source As Range
Set source = ActiveSheet.Range("A2:F6")
Set uniques = GetUniqueValues(source.Value)
Dim it
For Each it In uniques
Debug.Print it
Next
End Sub
Public Function GetUniqueValues(ByVal values As Variant) As Collection
Dim result As Collection
Dim cellValue As Variant
Dim cellValueTrimmed As String
Set result = New Collection
Set GetUniqueValues = result
On Error Resume Next
For Each cellValue In values
cellValueTrimmed = Trim(cellValue)
If cellValueTrimmed = "" Then GoTo NextValue
result.Add cellValueTrimmed, cellValueTrimmed
NextValue:
Next cellValue
On Error GoTo 0
End Function
Output
SGD
PHP
KRW
CNY
IDA
BGN
PDSS
CBBT
INPC
DBS
a
In case when the source range consists of areas get the values of all the areas first.
Public Function GetSourceValues(ByVal sourceRange As Range) As Collection
Dim vals As VBA.Collection
Dim area As Range
Dim val As Variant
Set vals = New VBA.Collection
For Each area In sourceRange.Areas
For Each val In area.Value
If val <> "" Then _
vals.Add val
Next val
Next area
Set GetSourceValues = vals
End Function
Source type is now Collection but then all works the same:
Dim uniques As Collection
Dim source As Collection
Set source = GetSourceValues(ActiveSheet.Range("A2:F6").SpecialCells(xlCellTypeVisible))
Set uniques = GetUniqueValues(source)