A more suitable and straightforward solution would be to use RecyclerView with GridLayoutManager. GridLayoutManager takes SpanSizeLookup object which allows you to specify how many spans will each of the items occupy.
A complete solution to this problem includes the following pieces:
Activity with RecyclerView, GridLayoutManager and custom SpanSizeLookup
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
RecyclerView recyclerView = (RecyclerView) findViewById(R.id.recycler_view);
// specify that grid will consist of 2 columns
GridLayoutManager gridLayoutManager = new GridLayoutManager(getApplicationContext(), 2);
// provide our CustomSpanSizeLookup which determines how many spans each item in grid will occupy
gridLayoutManager.setSpanSizeLookup(new CustomSpanSizeLookup());
// provide our GridLayoutManager to the view
recyclerView.setLayoutManager(gridLayoutManager);
// this is fake list of images
List<Integer> imageResList = getMockedImageList();
// finally, provide adapter to the recycler view
Adapter adapter = new Adapter(imageResList);
recyclerView.setAdapter(adapter);
}
private List<Integer> getMockedImageList() {
// fake images list, you'd need to upload your own image resources
List<Integer> imageResList = new ArrayList<Integer>();
imageResList.add(R.drawable.img1);
imageResList.add(R.drawable.img2);
imageResList.add(R.drawable.img3);
imageResList.add(R.drawable.img4);
imageResList.add(R.drawable.img5);
imageResList.add(R.drawable.img6);
return imageResList;
}
private static class CustomSpanSizeLookup extends GridLayoutManager.SpanSizeLookup {
@Override
public int getSpanSize(int i) {
if(i == 0 || i == 1) {
// grid items on positions 0 and 1 will occupy 2 spans of the grid
return 2;
} else {
// the rest of the items will behave normally and occupy only 1 span
return 1;
}
}
}
}
Adapter for RecyclerView
public class Adapter extends RecyclerView.Adapter {
// I assume that you will pass images as list of resources, but this can be easily switched to a list of URLS
private List<Integer> imageResList = new ArrayList<Integer>();
public Adapter(List<Integer> imageUrlList) {
this.imageResList = imageUrlList;
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup viewGroup, int i) {
View itemView = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.recycle_view_item, viewGroup, false);
return new ItemViewHolder(itemView);
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder viewHolder, int i) {
ItemViewHolder itemViewHolder = (ItemViewHolder) viewHolder;
itemViewHolder.item.setImageResource(imageResList.get(i));
}
@Override
public int getItemCount() {
return imageResList.size();
}
private static class ItemViewHolder extends RecyclerView.ViewHolder {
private ImageView item;
public ItemViewHolder(View itemView) {
super(itemView);
this.item = (ImageView) itemView.findViewById(R.id.item_image);
}
}
}
activity_main.xml layout
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</RelativeLayout>
recycler_view_item.xml layout
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="150dp"
android:padding="10dp">
<ImageView
android:id="@+id/item_image"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"/>
</LinearLayout>
And the last piece would be to make sure to add dependency in build.gradle file for RecyclerView:
dependencies {
compile 'com.android.support:recyclerview-v7:21.0.+'
}
And here is the result:
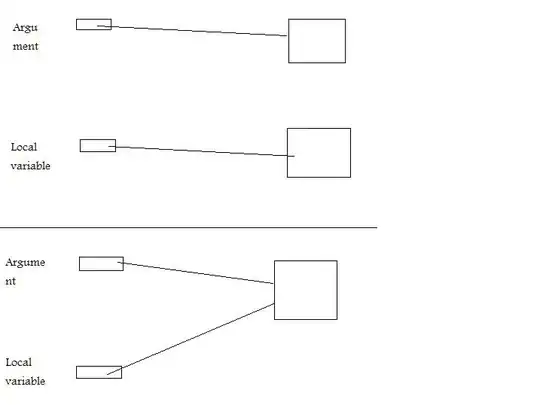
Advantages of this solution are:
- it's scalable, lightweight, and very customizable
- it's efficient as it recycles the views when you scroll up and down
- it does not require messing with touch events which can easily become very tricky to handle once you add any additional touch functionality
I hope this is helpful.