In VB.NET you can make your application single instance from the project properties page. Check the "Make single instance application" option, then click the "View Application Events" button:
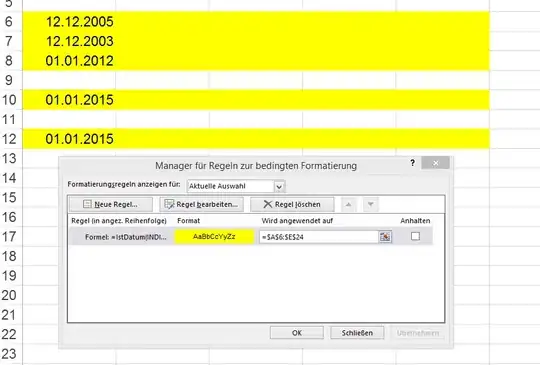
In the ApplicationEvents.vb
class, add a handler for StartupNextInstance
- this will be called when the application is already running and you start it again. You can call a method on your main form:
Namespace My
Partial Friend Class MyApplication
Private Sub MyApplication_StartupNextInstance(sender As Object, e As ApplicationServices.StartupNextInstanceEventArgs) Handles Me.StartupNextInstance
' Handle arguments when app is already running
If e.CommandLine.Count > 0 Then
' Pass the argument to the main form
Dim form = TryCast(My.Application.MainForm, Form1)
form.LoadFile(e.CommandLine(0))
End If
End Sub
End Class
End Namespace
In your main form, you can pass the initial command line arguments, and handle the subsequent ones, with a common method:
Public Class Form1
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles Me.Load
' Handle arguments from the initial launch
Dim args = Environment.GetCommandLineArgs()
If args.Length > 1 Then
LoadFile(args(1))
End If
End Sub
Public Sub LoadFile(filename As String)
MessageBox.Show(filename)
End Sub
End Class