Things to note:
- number of characters in rows/cols is n
- first row will always have n - 1
-
s and one \
- last row will always have n - 2
-
s, begin with \
and ends with /
For example, when n
is 4:
First row: - - - \
Last row: \ - - /
Can be easily achieved using:
def get_first_raw(n):
return '- ' * (n - 1) + '\\'
def get_last_raw(n):
return '\\ ' + '- ' * (n - 2) + '/'
Now regarding the body of the spiral, note the following:
For n = 3:
- - \
/ \ |
\ - /
For n = 5:
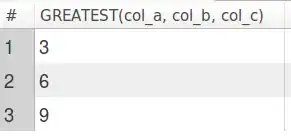
For n = 6:
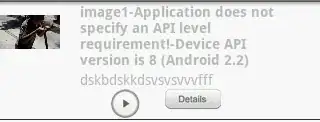
Note that the 4-spiral is contained inside it, and the red boxes are fixed. Only their length changes according to n.
It's contained inside it. And fore n = 7, the n = 5 is contained inside it. The same holds for n = 2k, each n will have n/2 spiral contained in it.
What I'm trying to say here that you manually draw n = 3 and n = 2. If the spiral should be made from an even number, you use the n = 2 pattern, construct the first and last rows, and using loops you can append the body of the spiral.
Example for n = 5:
def get_spiral(n):
res = []
res.append(get_first_raw(n))
res.append('/ ' + spiral[0] + ' |')
for line in spiral[1:]:
res.append('| ' + line + ' |')
res.append(get_last_raw(n))
return res
print '\n'.join(get_spiral(5))
where spiral
is an initial spiral of size 3:
spiral = ['- - \\', '/ \ |', '\ - /']
In order to generate 7-spiral, you do:
spiral = build_spiral(5)
print '\n'.join(build_spiral(7))
and you'll get:
- - - - - - \
/ - - - - \ |
| / - - \ | |
| | / \ | | |
| | \ - / | |
| \ - - - / |
\ - - - - - /
Of course this can be improved and you can make the program more efficient, I just wanted to give you a guideline and to share my thoughts..
Here's more spirals for fun:
- - - - - - - - - - \
/ - - - - - - - - \ |
| / - - - - - - \ | |
| | / - - - - \ | | |
| | | / - - \ | | | |
| | | | / \ | | | | |
| | | | \ - / | | | |
| | | \ - - - / | | |
| | \ - - - - - / | |
| \ - - - - - - - / |
\ - - - - - - - - - /
- - - - - - - - - - - - - - - - - - - - - - - - \
/ - - - - - - - - - - - - - - - - - - - - - - \ |
| / - - - - - - - - - - - - - - - - - - - - \ | |
| | / - - - - - - - - - - - - - - - - - - \ | | |
| | | / - - - - - - - - - - - - - - - - \ | | | |
| | | | / - - - - - - - - - - - - - - \ | | | | |
| | | | | / - - - - - - - - - - - - \ | | | | | |
| | | | | | / - - - - - - - - - - \ | | | | | | |
| | | | | | | / - - - - - - - - \ | | | | | | | |
| | | | | | | | / - - - - - - \ | | | | | | | | |
| | | | | | | | | / - - - - \ | | | | | | | | | |
| | | | | | | | | | / - - \ | | | | | | | | | | |
| | | | | | | | | | | / \ | | | | | | | | | | | |
| | | | | | | | | | | \ - / | | | | | | | | | | |
| | | | | | | | | | \ - - - / | | | | | | | | | |
| | | | | | | | | \ - - - - - / | | | | | | | | |
| | | | | | | | \ - - - - - - - / | | | | | | | |
| | | | | | | \ - - - - - - - - - / | | | | | | |
| | | | | | \ - - - - - - - - - - - / | | | | | |
| | | | | \ - - - - - - - - - - - - - / | | | | |
| | | | \ - - - - - - - - - - - - - - - / | | | |
| | | \ - - - - - - - - - - - - - - - - - / | | |
| | \ - - - - - - - - - - - - - - - - - - - / | |
| \ - - - - - - - - - - - - - - - - - - - - - / |
\ - - - - - - - - - - - - - - - - - - - - - - - /
You also got a top view for a pyramid for free