Save the context, add a clip, set the scale, and draw the image.
This should be close:
circlePath.lineCapStyle = kCGLineCapRound; // existing
// add these lines
CGContextSaveGState(context)
circlePath.addClip()
CGContextScaleCTM(context, 1, -1)
CGContextDrawTiledImage(context, CGRectMake(0, 0, test-shape.size.width, test-shape.size.height), test-shape.CGImage)
CGContextRestoreGState(context)
UIColor.grayColor().setStroke() //existing
Edit to add alternative: This draws a donut and fills it with the image. But the line dash is not probably going to have the effect you need. Perhaps this can help in some way.
let context = UIGraphicsGetCurrentContext()
let testshape = UIImage(named: "testshape.png")!
var circlePath = UIBezierPath()
circlePath.moveToPoint(CGPointMake(9.5, 2.5))
circlePath.addCurveToPoint(CGPointMake(6.1, 3.38), controlPoint1: CGPointMake(8.27, 2.5), controlPoint2: CGPointMake(7.11, 2.82))
circlePath.addCurveToPoint(CGPointMake(2.5, 9.5), controlPoint1: CGPointMake(3.95, 4.58), controlPoint2: CGPointMake(2.5, 6.87))
circlePath.addCurveToPoint(CGPointMake(9.5, 16.5), controlPoint1: CGPointMake(2.5, 13.37), controlPoint2: CGPointMake(5.63, 16.5))
circlePath.addCurveToPoint(CGPointMake(16.5, 9.5), controlPoint1: CGPointMake(13.37, 16.5), controlPoint2: CGPointMake(16.5, 13.37))
circlePath.addCurveToPoint(CGPointMake(9.5, 2.5), controlPoint1: CGPointMake(16.5, 5.63), controlPoint2: CGPointMake(13.37, 2.5))
circlePath.closePath()
circlePath.moveToPoint(CGPointMake(19, 9.5))
circlePath.addCurveToPoint(CGPointMake(9.5, 19), controlPoint1: CGPointMake(19, 14.75), controlPoint2: CGPointMake(14.75, 19))
circlePath.addCurveToPoint(CGPointMake(0, 9.5), controlPoint1: CGPointMake(4.25, 19), controlPoint2: CGPointMake(-0, 14.75))
circlePath.addCurveToPoint(CGPointMake(3.88, 1.84), controlPoint1: CGPointMake(0, 6.36), controlPoint2: CGPointMake(1.53, 3.57))
circlePath.addCurveToPoint(CGPointMake(9.5, 0), controlPoint1: CGPointMake(5.45, 0.68), controlPoint2: CGPointMake(7.4, 0))
circlePath.addCurveToPoint(CGPointMake(19, 9.5), controlPoint1: CGPointMake(14.75, 0), controlPoint2: CGPointMake(19, 4.25))
circlePath.closePath()
circlePath.lineCapStyle = kCGLineCapRound;
CGContextSaveGState(context)
circlePath.addClip()
CGContextScaleCTM(context, 1, -1)
CGContextDrawTiledImage(context, CGRectMake(0, 0, testshape.size.width, testshape.size.height), testshape.CGImage)
CGContextRestoreGState(context)
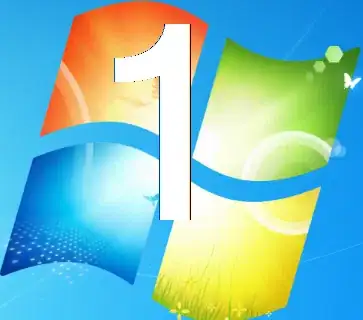
If you just want a half circle, here is a quick example of how to draw that. Just use that as the mask. Rotate as needed.
let color = UIColor(red: 0.742, green: 0.000, blue: 1.000, alpha: 1.000)
var bezierPath = UIBezierPath()
bezierPath.moveToPoint(CGPointMake(56, 45))
bezierPath.addCurveToPoint(CGPointMake(31, 70), controlPoint1: CGPointMake(56, 58.81), controlPoint2: CGPointMake(44.81, 70))
bezierPath.addCurveToPoint(CGPointMake(26, 65), controlPoint1: CGPointMake(28.24, 70), controlPoint2: CGPointMake(26, 67.76))
bezierPath.addCurveToPoint(CGPointMake(31, 60), controlPoint1: CGPointMake(26, 62.24), controlPoint2: CGPointMake(28.24, 60))
bezierPath.addCurveToPoint(CGPointMake(46, 45), controlPoint1: CGPointMake(39.28, 60), controlPoint2: CGPointMake(46, 53.28))
bezierPath.addCurveToPoint(CGPointMake(31, 30), controlPoint1: CGPointMake(46, 36.72), controlPoint2: CGPointMake(39.28, 30))
bezierPath.addCurveToPoint(CGPointMake(26, 25), controlPoint1: CGPointMake(28.24, 30), controlPoint2: CGPointMake(26, 27.76))
bezierPath.addCurveToPoint(CGPointMake(29.45, 20.24), controlPoint1: CGPointMake(26, 22.78), controlPoint2: CGPointMake(27.45, 20.9))
bezierPath.addCurveToPoint(CGPointMake(31, 20), controlPoint1: CGPointMake(29.94, 20.09), controlPoint2: CGPointMake(30.46, 20))
bezierPath.addCurveToPoint(CGPointMake(56, 45), controlPoint1: CGPointMake(44.81, 20), controlPoint2: CGPointMake(56, 31.19))
bezierPath.closePath()
color.setFill()
bezierPath.fill()
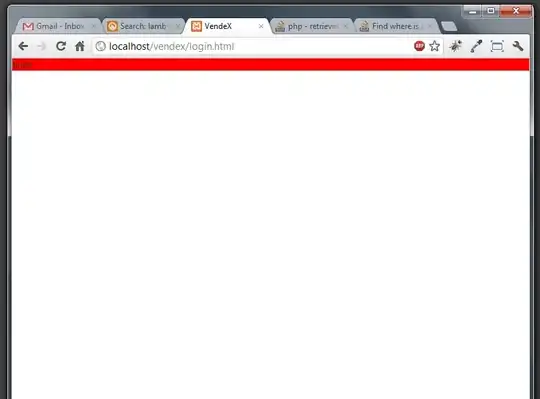