This is how I usually setup my code if I want to have a fine control over the size of the figure's margins in matplotlib. In addition, I show how the position of the ylabel can be setup, so you can easily align the ylabels of your two figures together.
import matplotlib.pyplot as plt
plt.close('all')
#---- create figure ----
fwidth = 8. # total width of the figure in inches
fheight = 4. # total height of the figure in inches
fig = plt.figure(figsize=(fwidth, fheight))
#---- define margins -> size in inches / figure dimension ----
left_margin = 0.95 / fwidth
right_margin = 0.2 / fwidth
bottom_margin = 0.5 / fheight
top_margin = 0.25 / fheight
#---- create axes ----
# dimensions are calculated relative to the figure size
x = left_margin # horiz. position of bottom-left corner
y = bottom_margin # vert. position of bottom-left corner
w = 1 - (left_margin + right_margin) # width of axes
h = 1 - (bottom_margin + top_margin) # height of axes
ax = fig.add_axes([x, y, w, h])
#---- Define the Ylabel position ----
# Location are defined in dimension relative to the figure size
xloc = 0.25 / fwidth
yloc = y + h / 2.
ax.set_ylabel('yLabel', fontsize=16, verticalalignment='top',
horizontalalignment='center')
ax.yaxis.set_label_coords(xloc, yloc, transform = fig.transFigure)
plt.show(block=False)
fig.savefig('figure_margins.png')
This results in a 8in x 4in figure, with margins of exactly 0.95, 0.2, 0.5, and 0.25 inch at the left, right, bottom and top of the figure. One benefit of this approach is that the size of the margins are defined in absolute units (inches), meaning they will remain consistent even if you change the size of the figure.
As for the ylabel, horizontally, the top of the label is located 0.25 inch from the left edge of the figure, while vertically the centre of the label corresponds to the centre of the axe. Note that due to the 90 degrees rotation on the ylabel, the meaning of the verticalalignment
and horizontalalignment
are in reality inverted.
Below are shown outputs of the code above with the yaxis limits set to [0, 1] and to [0, 18] respectively.
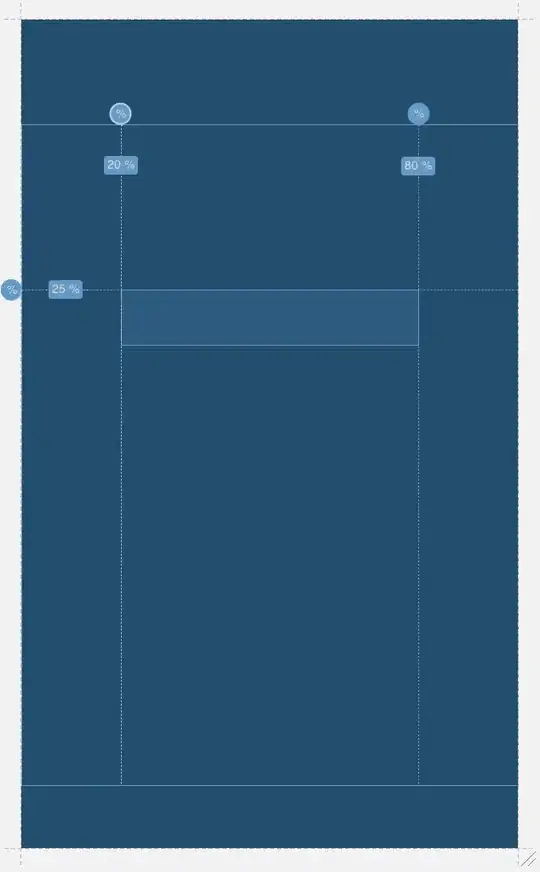