This code is equivalent to:
#include <stdio.h>
int main(void) {
char direction_name = 1["nsew"];
printf("[%c]\n",direction_name);
return 0;
}
which will print [s], because
char direction_name = 1["nsew"]; // "nsew" is string literal, i.e. an array
// of 5 chars 'n' 's' 'e' 'w' '\0'
is equal to
char direction_name = "nsew"[1];
and also to
char direction_name = *("nsew" +1);
Therefore []
is symmetric in a sense that
x[y] == y[x] // if conditions given in C standard 6.5.2.1/2 are met
You can think of [] as an symmetric relation in algebra (under assumptions from 6.5.2.1 § 2):
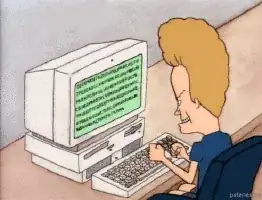
Or you may think about [] as a linear mapping (transformation, functional) between reflexive (Banach) linear spaces V and V** if you wish:
x[f] = [f,x] = f[x]
C standard n1124 6.5.2.1 § 2 Array subscripting (emphasis main)
A postfix expression followed by an expression in square brackets []
is a subscripted designation of an element of an array object. The definition of the subscript operator []
is that E1[E2]
is identical to (*((E1)+(E2)))
. Because of the conversion rules that apply to the binary + operator, if E1
is an array object (equivalently, a pointer to the initial element of an array object) and E2
is an integer, E1[E2]
designates the E2
-th element of E1
(counting from zero).