first thing is that ALL ModelViewSet urls have names, even if you don´t set those names explicitly.
You can find out how the default urls are created within a router, in the docs: http://www.django-rest-framework.org/api-guide/routers/ (see 'SimpleRouter' url names table)
To see all the actual url names available in your application, try with this utility:
def print_url_pattern_names(patterns):
"""Print a list of urlpattern and their names"""
for pat in patterns:
if pat.__class__.__name__ == 'RegexURLResolver': # load patterns from this RegexURLResolver
print_url_pattern_names(pat.url_patterns)
elif pat.__class__.__name__ == 'RegexURLPattern': # load name from this RegexURLPattern
if pat.name is not None:
print '[API-URL] {} \t\t\t-> {}'.format(pat.name, pat.regex.pattern)
Then, in your urls.py:
urlpatterns = [
url(r'^', include(router.urls)),
]
if settings.DEBUG:
print_url_pattern_names(urlpatterns)
If you want the url to be different from your ModelViewSet´s name (as in my case) you can set it on the router with "base_name":
router.register('contents', media_views.MediaViewSet, base_name='contents')
Next thing you´ll need is extending HyperlinkedModelSerializer:
from rest_framework import serializers
# in this sample my object is of type "Media"
class MediaSerializer(serializers.HyperlinkedModelSerializer):
#blablabla
You will have a serializer ready to show hyperlinks to your detail view, but there is one required step left for this to work. That´s where the "view_name" comes in:
from rest_framework import serializers
class MediaSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = Media
fields = ('url', ...[other fields you want to serialize])
extra_kwargs = {
'url': {'view_name': 'contents-detail'}
}
- 'url' field is mandatory to show the link.
- With 'extra_kwargs' > view_name you´re telling the framework that your detail view´s name is 'content-detail'.
- 'content-detail' is just MY view´s name. You
need to find out yours (from the url names utility above)
And this is the http response from the sample (see url field):
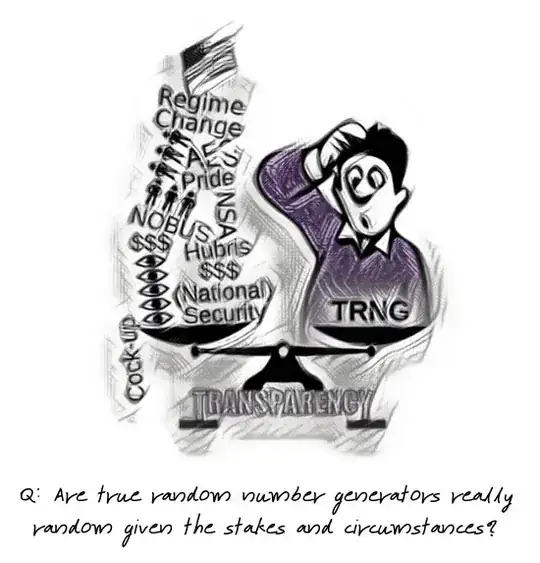