You can use minEnclosingCircle to find the smallest circle containing all your points.
You get the center
as output value of the function:
void minEnclosingCircle(InputArray points, Point2f& center, float& radius)
UPDATE
I tried a few different things. I assumed that you know that your final shape would be a circle.
minEnclosingCircle
boundingRect
fitEllipse
The best result (in this image) seems to be with fitEllipse
.
Results
minEnclosingCircle:
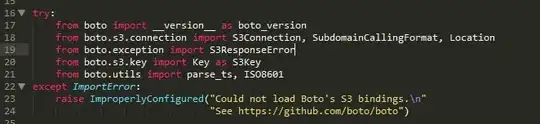
boundingRect:
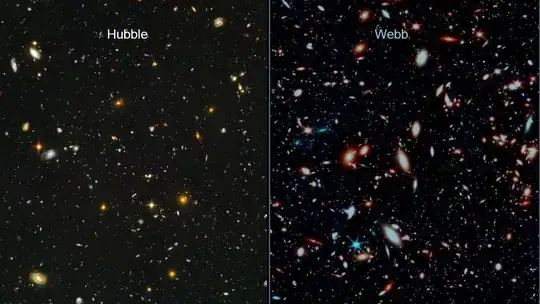
fitEllipse:
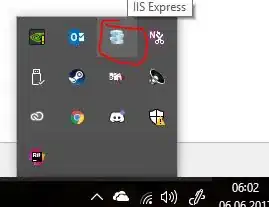
Code:
#include <opencv2\opencv.hpp>
#include <vector>
using namespace std;
using namespace cv;
int main()
{
Mat1b img = imread("path_to_image", IMREAD_GRAYSCALE);
vector<Point> points;
findNonZero(img, points);
Mat3b res;
cvtColor(img, res, CV_GRAY2BGR);
//////////////////////////////////
// Method 1: minEnclosingCircle
//////////////////////////////////
/*Point2f center;
float radius;
minEnclosingCircle(points, center, radius);
circle(res, center, radius, Scalar(255,0,0), 1);
circle(res, center, 5, Scalar(0,255,0), 1);*/
//////////////////////////////////
// Method 2: boundingRect
//////////////////////////////////
/*Rect bbox = boundingRect(points);
rectangle(res, bbox, Scalar(0,255,255));
circle(res, Point(bbox.x + bbox.width/2, bbox.y + bbox.height/2), 5, Scalar(0,0,255));*/
//////////////////////////////////
// Method 3: fit ellipse
//////////////////////////////////
RotatedRect ell = fitEllipse(points);
ellipse(res, ell, Scalar(255,255,0));
circle(res, ell.center, 5, Scalar(255,0,255));
return 0;
}