Here is the solution:
First, we need to make us familiar with the Winapi FILETIME structure.
Then get the IE cookie files from here:
C:\Users\%username%\AppData\Roaming\Microsoft\Windows\Cookies\
Now look for the cookie files with the identical name=value
pairs you got from TEmbeddedWB.Cookie
.
Here is an example of the content of a IE cookie file, where we take our data from:
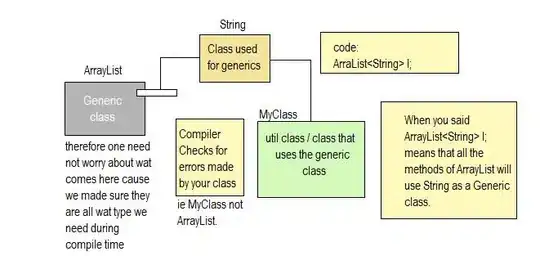
(Similarities with people alive or other authorities are purely accidental and not intended!)
We can see the meaning of the various numbers from the red colored comments.
And here is the source code to decipher those numbers:
uses Winapi.Windows;
function ConvertWinapiFileTimeLoHiValuesToDateTimeStr(const AFTLoValue, AFTHiValue: Cardinal): string;
const
InvalidDate = '01/01/80 12:00:00 AM';
var
lCookieFileTime: TFileTime;
lDosDT: Integer;
lLocalFileTime: TFileTime;
begin
lCookieFileTime.dwLowDateTime := AFTLoValue;
lCookieFileTime.dwHighDateTime := AFTHiValue;
FileTimeToLocalFiletime(lCookieFileTime, lLocalFileTime);
if FileTimeToDosDateTime(lLocalFileTime, Longrec(lDosDT).Hi, Longrec(lDosDT).Lo) then
begin
try
Result := DateTimeToStr(FiledateToDatetime(lDosDT));
except
Result := InvalidDate;
end;
end
else
Result := InvalidDate;
end;
Now we can use this function with the numbers from the above cookie file, as an example:
CodeSite.Send('Expiration Date', ConvertWinapiFileTimeLoHiValuesToDateTimeStr(2496134912, 30471078));
CodeSite.Send('Modified Date', ConvertWinapiFileTimeLoHiValuesToDateTimeStr(2624224682, 30465043));
Which will give us this result:
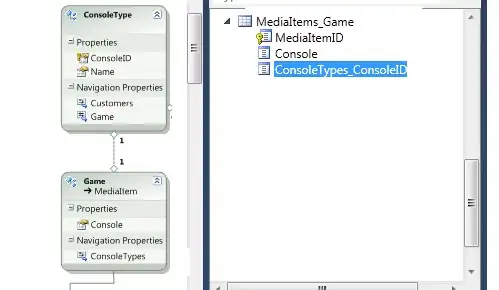