Construct a set for both rectangle and polygon and calculate the symmetric difference (vertices in either rectangle or polygon but not in both), for example:
rectangle = [(0, 0), (13, 0), (13, 10), (0, 10)]
polygon = [(0, 5), (0, 10), (2, 6), (8, 6), (11, 0), (13, 0), (13, 10)]
# "^" is the symmetric difference operator in python
set(rectangle) ^ (set(polygon))
Returns:
set([(11, 0), (2, 6), (0, 5), (0, 0), (8, 6)])
Which corresponds to the green area (vertices A, I, E, H, J) in the following picture:
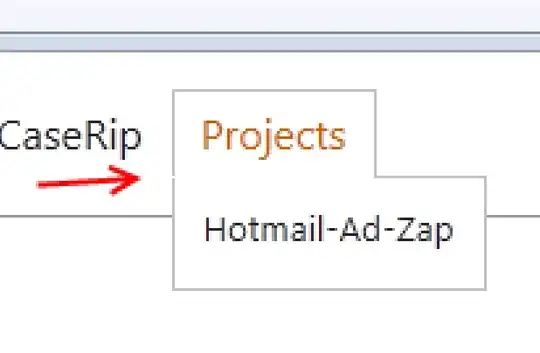
Beware that it will get the complement of the red polygon which does not include the intersection with the wall in your original image:
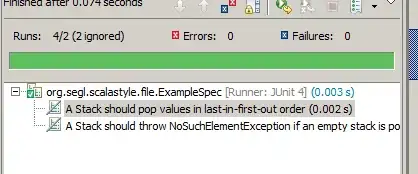
If you want your result to be the yellow polygon of the next figure instead:

Then you will have to do a rectangle-polygon intersection for every wall/block in the stage with the complement polygon using some method like the ones described in the following question:
Method to detect intersection between a rectangle and a polygon?