One possibility is to simply increment each index value by 1 (which changes the index in-place).
df = pd.DataFrame({'col': [1, 2, 3]})
df.index += 1
Another is to assign a new range index, that starts from 1, using set_axis()
.
df = pd.DataFrame({'col': [1, 2, 3]})
df = df.set_axis(range(1, len(df)+1))
In fact, since set_axis()
assigns a new object to the index, i.e. resets the index, it can be used instead of reset_index()
.
It is especially useful if you need to make the index to start from 1 in a pipeline (where assigning or incrementing index wouldn't work).
df = pd.DataFrame({'col': [4, 1, 2, 3]})
df = (
df
.reset_index()
.set_axis(range(1, len(df)+1))
)
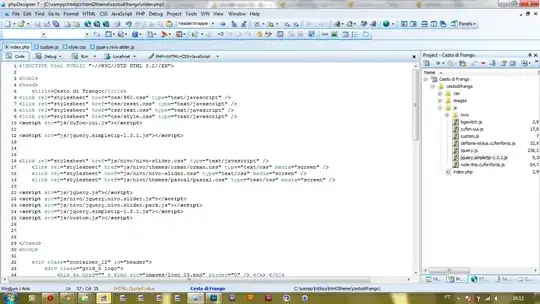
or the dataframe shape needs to be modified in the pipeline (e.g. using query()
), pipe()
could be used.
df = pd.DataFrame({'col': [4, 1, 2, 3]})
df = (
df
.query('col > 2')
.pipe(lambda x: x.set_axis(range(1, len(x)+1)))
)
