Its unclear exactly what you are asking. Do you want the repeated X
values to plot on top of each other? In which case, you can use ax.plot
as shown in the first example (ax1
) below.
If you want the X axis to show all the X
values in the order they appear in your list, you could use scatter, then just set the xticklabels
to the values in X
, as shown in the second example below (ax2
)
As stated above in comments, neither of these are particularly difficult: there are lots of examples in the matplotlib
gallery.
import matplotlib.pyplot as plt
X = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 100, 90, 80, 70, 60, 50, 40, 30, 20, 10]
Y1 = [0, 100, 200, 300, 400, 500, 600, 700, 800, 900, 900, 800, 700, 600, 500, 400, 300, 200, 100, 0]
Y2 = [100, 200, 300, 400, 500, 600, 700, 800, 900, 1000, 1000, 900, 800, 700, 600, 500, 400, 300, 200, 100]
fig = plt.figure()
ax1=plt.subplot(211)
ax2=plt.subplot(212)
# First example: overlap repeated X values
ax1.plot(X,Y1,'ro')
ax1.plot(X,Y2,'bo')
ax1.set_title('ax.plot')
# Second example: keep all X values in sequential order
ax2.scatter(range(len(Y1)),Y1,color='r')
ax2.scatter(range(len(Y2)),Y2,c='b')
ax2.set_title('ax.scatter')
ax2.set_xlim(0,len(Y1)-1)
ax2.set_xticks(range(len(Y1)))
ax2.set_xticklabels(X)
plt.show()
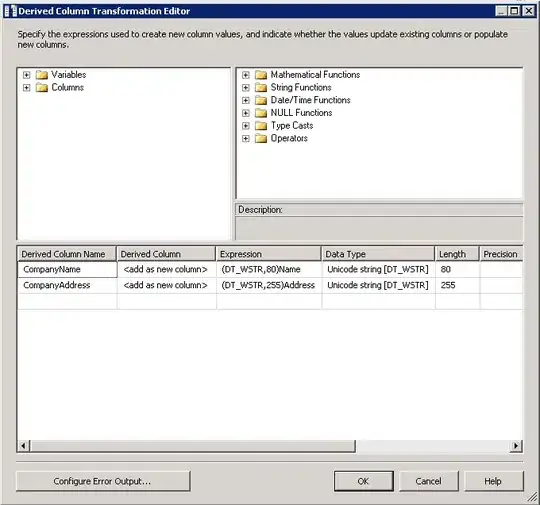