You can use the interp1d
function from scipy to create a linear interpolation between each set of points. interp1d
takes x and y values and returns a function that takes an x value and returns the corresponding y value. Once you have a function for stress and for y, you need to figure out where those intersect. One way to do this efficiently is with something like the bisection method, which finds zeros.
Here's the code: import numpy as np
import matplotlib.pyplot as plt
from scipy.interpolate import interp1d
from scipy.optimize import bisect
data = '''0 0
0.1 0.1
0.2 0.2
0.3 0.3
0.4 0.31
0.5 0.32
0.6 0.35'''
data = [line.split() for line in data.split('\n')]
data = np.array(data, dtype = 'float')
strain = data[:,0]
stress = data[:,1]
E = 1
y = [0, 0.5]
x = 0.2, ((y[1] - y[0])/E+0.2)
#use interp1d to get interpolated points
y = interp1d(x, y)
stress = interp1d(strain, stress)
#set starting points
x1 = max(x[0], strain[0])
x2 = min(x[-1], strain[-1])
max_err = .01
#create function
f = lambda x : stress(x) - y(x)
#find x1 where f(x1) = 0
x1 = bisect(f, x1, x2, xtol = .001)
y1 = stress(x1)
plt.figure(num=None, figsize=(10, 6), dpi=100, facecolor='w', edgecolor='k')
plt.plot(strain, stress(strain))
plt.plot(x, y(x))
plt.scatter(x1, y1)
plt.show()
And the resulting image: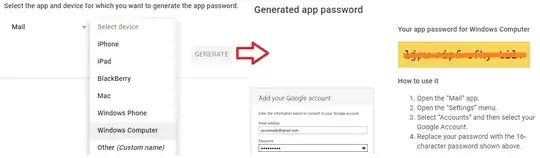