You are not using arrays (nor JSON) but objects.
If you want to merge two objets, one solution is to use next Javascript API (ES6) Object.assign
method through a polyfill (polyfills give you the ability to use standard APIs that are not yet supported):
var obj1 = {
id: 1,
Oid: 'ff2d344e-77e8-43b3-a6f3-bfba577d6abd',
name: 'Some name'
}
var obj2 = {
id: 3,
array_ref: 'ff2d344e-77e8-43b3-a6f3-bfba577d6abd',
value1: 'Additional Data',
value2: 'Some other additional data'
}
var obj3 = Object.assign({}, obj1, obj2);
console.log(obj3);
<script src="https://cdnjs.cloudflare.com/ajax/libs/es6-shim/0.33.3/es6-shim.js"></script>
Which will gives you:
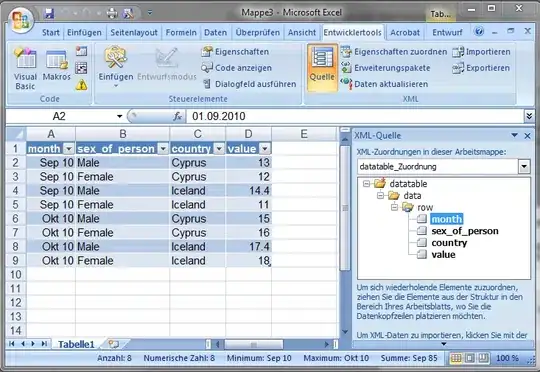
In the above snippet I included a whole es6 polyfill but this polyfill here only contains what you are looking for and is probably better if you want to avoid loading a whole bunch of useless code (I could not easily load it in the snippet).
If you use jQuery or underscore, they also both provides an extend
method that you can use instead of a polyfill.
Warning! As you can see, this will actually overwrites any content in obj1
that is also in obj2
with the content of obj2
. Here, for example, the resulting object has the id
of obj2
. If you are looking for a more clever kind of merging, you will need to go for more complicated algorithmic.
If you want to merge only the objects with the same id, I would use underscore:
var objectList = [
{ id: 1, val: "val1", otherVal: "val2" },
{ id: 2, val: "val3" },
{ id: 1, val: "val4", differentVal: "val5" }
];
// group objects by ids
var groups = _.groupBy(objectList, 'id');
// merge the groups
var mergedObjects = _.map(groups, function(objects){
// Adds a new empty object at the beginning of the list
// to avoid modifying the original objects.
// It will be the destination of _.extend.
objects.unshift({});
// use extend with apply so we can provide it an array to be used as arguments
return _.extend.apply(_, objects);
});
console.log(mergedObjects);
<script src="http://underscorejs.org/underscore-min.js"></script>
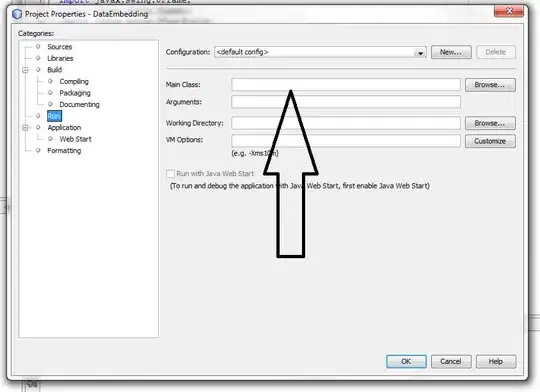
Notice that some overwriting still can happen (first object's val
is overwritten by third object's val
here).
Edit: As pointed out in the comment, lodash is likely to be a better solution than underscore. Read here about lodash vs. underscore.