If you want use <span>
element inside of the custom cell formatter you can return from the custom formatter
return '<span class="cellWithoutBackground" style="background-color:' +
color + ';">' + cellvalue + '</span>';
where the style of span.cellWithoutBackground
you can define for example like following
span.cellWithoutBackground
{
display:block;
background-image:none;
margin-right:-2px;
margin-left:-2px;
height:14px;
padding:4px;
}
How it works you can see live here:
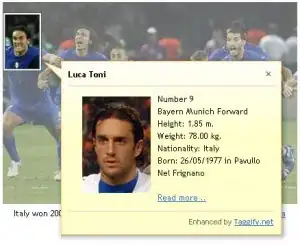
UPDATED: The answer is old. The best practice would be to use cellattr
callback in colModel
instead of the usage custom formatters. Changing of background color of the cell is in general just assigning style
or class
attribute to the cells of the column (<td>
elements). The cellattr
callback defined in the column of colModel
allows exactly to do this. One can still use predefined formatters like formatter: "checkbox"
, formatter: "currency"
, formatter: "date"
and so on, but still change the background color in the column. In the same way the rowattr
callback, which can be defined as the jqGrid option (outside of specific column of colModel
), allows to assign style/class of the whole row (<tr>
elements).
More information about cellattr
can be found here and here, for example. Another answer explains rowattr
.