This is doable by providing a dummy select item and explicitly checking for it in the ajax change listener. Once selected, just show the dialog. When the dialog is saved, then save the new job and reset the dummy select item.
Here's a kickoff example.
@Named
@ViewScoped
public class Bean implements Serializable {
private List<Activity> activities;
private List<Job> jobs;
private Job newJob;
@EJB
private YourActivityService yourActivityService;
@EJB
private YourJobService yourJobService;
@PostConstruct
public void init() {
activities = yourActivityService.list();
jobs = yourJobService.list();
newJob = new Job();
}
public void addNewJobIfNecessary(AjaxBehaviorEvent event) {
if (newJob.equals(((UIInput) event.getComponent()).getValue())) {
RequestContext ajax = RequestContext.getCurrentInstance();
ajax.update("addNewJobDialog");
ajax.execute("PF('widget_addNewJobDialog').show()");
}
}
public void saveNewJob() {
yourJobService.save(newJob);
jobs.add(newJob);
newJob = new Job();
RequestContext ajax = RequestContext.getCurrentInstance();
ajax.update("activitiesForm");
ajax.execute("PF('widget_addNewJobDialog').hide()");
}
// ...
}
<h:form id="activitiesForm">
<p:dataTable value="#{bean.activities}" var="activity">
<p:column>#{activity.id}</p:column>
<p:column>
<p:selectOneMenu value="#{activity.job}" converter="omnifaces.SelectItemsConverter">
<f:selectItem itemValue="#{null}" itemLabel="Select one" />
<f:selectItems value="#{bean.jobs}" />
<f:selectItem itemValue="#{bean.newJob}" itemLabel="Add new Job" />
<p:ajax listener="#{bean.addNewJobIfNecessary}" />
</p:selectOneMenu>
</p:column>
</p:dataTable>
</h:form>
<p:dialog id="addNewJobDialog">
<h:form>
<p:inputText value="#{bean.newJob.name}" required="true" />
<p:commandButton value="Add" action="#{bean.saveNewJob}" update="@form" />
<p:messages />
</h:form>
</p:dialog>

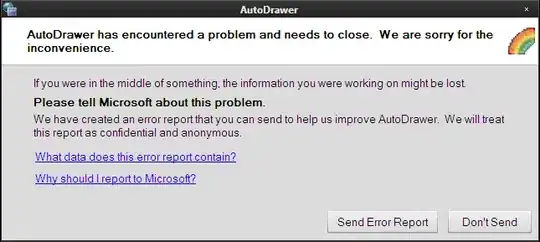
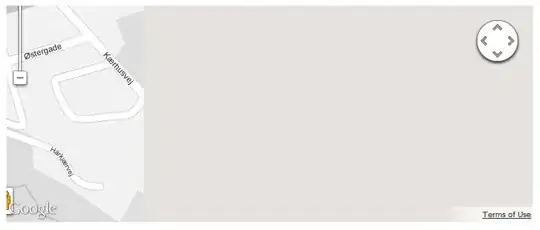

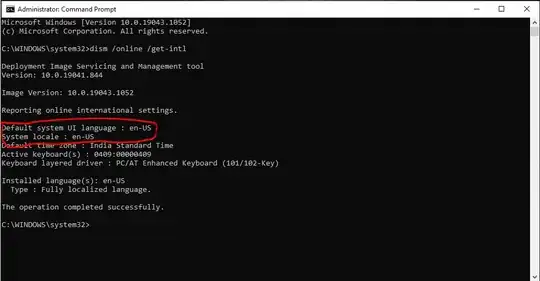