Try this. This uses Array to do the entire operation. This is very fast as compared to looping through every range.
Logic:
- Loop through the sheets and find the last row and last column
- Identify the range instead of using
UsedRange
blindly. You may want to see THIS
- Copy that data into array
- Clearing the Sheet - Resets the format of the sheet to
General
. Hope you have no other formatting in the sheet? If you have then see second piece of code.
- Paste the data back to the sheet.
Code
Sub Sample()
Dim ws As Worksheet
Dim usdRng As Range
Dim lastrow As Long, lastcol As Long
Dim myAr
For Each ws In Sheets
With ws
'~~> Check if sheet has any data
If Application.WorksheetFunction.CountA(.Cells) <> 0 Then
'~~> Find Last Row
lastrow = .Cells.Find(What:="*", _
After:=.Range("A1"), _
Lookat:=xlPart, _
LookIn:=xlFormulas, _
SearchOrder:=xlByRows, _
SearchDirection:=xlPrevious, _
MatchCase:=False).Row
'~~> Find last column
lastcol = .Cells.Find(What:="*", _
After:=.Range("A1"), _
Lookat:=xlPart, _
LookIn:=xlFormulas, _
SearchOrder:=xlByColumns, _
SearchDirection:=xlPrevious, _
MatchCase:=False).Column
'~~> Set your range here
Set usdRng = .Range("A1:" & _
Split(.Cells(, lastcol).Address, "$")(1) & lastrow)
'~~> Write to array
myAr = usdRng.Value
'~~> Clear the sheet
.Cells.Clear
'~~> Write back to the sheet
.Range("A1").Resize(lastrow, lastcol).Value = myAr
End If
End With
Next
End Sub
ScreenShot
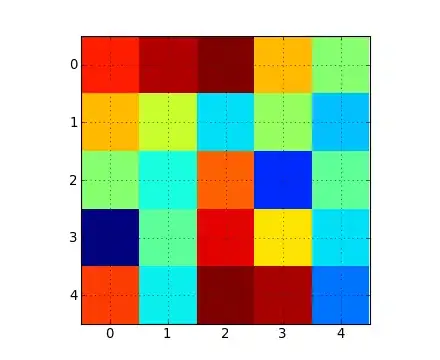
EDIT
If you have other formats in the sheet then use this
Sub Sample()
Dim ws As Worksheet
Dim usdRng As Range, rng as Range
Dim lastrow As Long, lastcol As Long
Dim myAr
For Each ws In Sheets
With ws
'~~> Check if sheet has any data
If Application.WorksheetFunction.CountA(.Cells) <> 0 Then
'~~> Find Last Row
lastrow = .Cells.Find(What:="*", _
After:=.Range("A1"), _
Lookat:=xlPart, _
LookIn:=xlFormulas, _
SearchOrder:=xlByRows, _
SearchDirection:=xlPrevious, _
MatchCase:=False).Row
'~~> Find last column
lastcol = .Cells.Find(What:="*", _
After:=.Range("A1"), _
Lookat:=xlPart, _
LookIn:=xlFormulas, _
SearchOrder:=xlByColumns, _
SearchDirection:=xlPrevious, _
MatchCase:=False).Column
'~~> Set your range here
Set usdRng = .Range("A1:" & _
Split(.Cells(, lastcol).Address, "$")(1) & lastrow)
On Error Resume Next
Set rng = usdRng.SpecialCells(xlCellTypeConstants)
On Error GoTo 0
If Not rng Is Nothing Then
'~~> Write to array
myAr = usdRng.Value
'~~> Clear the Range
rng.NumberFormat = "0.00"
Set rng = Nothing
'~~> Clear contents of the sheet
usdRng.ClearContents
'~~> Write back to the sheet
.Range("A1").Resize(lastrow, lastcol).Value = myAr
End If
End If
End With
Next
End Sub
Screenshot
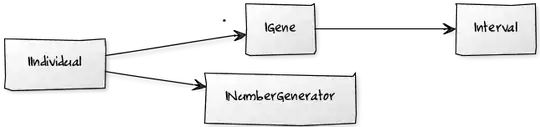