Well, a two-step story to get 'em PLOT really nice
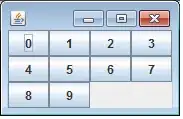
step 1: from a string
to a datetime
instance
step 2: from a datetime
to a matplotlib
convention compatible float
for dates/times
As usual, devil is hidden in detail.
matplotlib
dates are almost equal, but not equal:
# mPlotDATEs.date2num.__doc__
#
# *d* is either a class `datetime` instance or a sequence of datetimes.
#
# Return value is a floating point number (or sequence of floats)
# which gives the number of days (fraction part represents hours,
# minutes, seconds) since 0001-01-01 00:00:00 UTC, *plus* *one*.
# The addition of one here is a historical artifact. Also, note
# that the Gregorian calendar is assumed; this is not universal
# practice. For details, see the module docstring.
So, highly recommended to re-use their "own" tool:
from matplotlib import dates as mPlotDATEs # helper functions num2date()
# # and date2num()
# # to convert to/from.
Managing axis-labels & formatting & scale (min/max) is a separate issue
Nevertheless, matplotlib brings you arms for this part too:
from matplotlib.dates import DateFormatter, \
AutoDateLocator, \
HourLocator, \
MinuteLocator, \
epoch2num
from matplotlib.ticker import ScalarFormatter, FuncFormatter
and may for example do:
aPlotAX.set_xlim( x_min, x_MAX ) # X-AXIS LIMITs ------------------------------------------------------------------------------- X-LIMITs
#lt.gca().xaxis.set_major_locator( matplotlib.ticker.FixedLocator( secs ) )
#lt.gca().xaxis.set_major_formatter( matplotlib.ticker.FuncFormatter( lambda pos, _: time.strftime( "%d-%m-%Y %H:%M:%S", time.localtime( pos ) ) ) )
aPlotAX.xaxis.set_major_locator( AutoDateLocator() )
aPlotAX.xaxis.set_major_formatter( DateFormatter( '%Y-%m-%d %H:%M' ) ) # ----------------------------------------------------------------------------------------- X-FORMAT
#--------------------------------------------- # 90-deg x-tick-LABELs
plt.setp( plt.gca().get_xticklabels(), rotation = 90,
horizontalalignment = 'right'
)
#------------------------------------------------------------------