First reshape you data to long format:
library(reshape2)
x <- melt(df)
names(x) <- c("iplan","vplan","value")
After that you can easily make a plot with for example geom_point
:
library(ggplot2)
ggplot(x, aes(iplan, vplan)) +
geom_point(aes(size = value), alpha=0.8, color="darkgreen", show_guide=FALSE) +
geom_text(aes(label = value), color="white") +
scale_size(range = c(15,50)) +
theme_bw()
this gives:
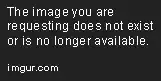
As an alternative, you could also consider geom_tile
:
ggplot(x, aes(iplan, vplan)) +
geom_tile(aes(fill = value)) +
geom_text(aes(label = value), color="white") +
scale_x_discrete(expand = c(0,0)) +
scale_y_discrete(expand = c(0,0)) +
scale_fill_gradient("Legend label", low = "lightblue", high = "blue") +
theme_bw()
wich gives:

Used data:
df <- structure(list(iplan = structure(1:2, .Label = c("No", "Yes"), class = "factor"), No = c(27L, 243L), Yes = c(239L, 2159L)), .Names = c("iplan", "No", "Yes"), class = "data.frame", row.names = c(NA, -2L))