Update 2
So I added to the ConfigBox.java
source code and made jButton1
open home\doc\READTHIS.txt
in Notepad. I created an executable jar
and the execution of the jar
, via java -jar Racercraft.jar
, is shown in the image below. Just take the example of what I did in ConfigBox.java
and apply it to NumberAdditionUI.java
for each of its JButtons
, making sure to change the filePath
variable to the corresponding file name that you would like to open.
Note: The contents of the JTextArea
in the image below were changed during testing, my code below does not change the contents of the JTextArea
.
Directory structure:
\home
Rasercraft.jar
\docs
READTHIS.txt
Code:
// imports and other code left out
public class ConfigBox extends javax.swing.JFrame {
// curDir will hold the absolute path to 'home\'
String curDir; // add this line
/**
* Creates new form ConfigBox
*/
public ConfigBox()
{
// this is where curDir gets set to the absolute path of 'home/'
curDir = new File("").getAbsolutePath(); // add this line
initComponents();
}
/*
* irrelevant code
*/
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed
Runtime rt = Runtime.getRuntime();
// filePath is set to 'home\docs\READTHIS.txt'
String filePath = curDir + "\\docs\\READTHIS.txt"; // add this line
try {
Process p = rt.exec("notepad " + filePath); // add filePath
} catch (IOException ex) {
Logger.getLogger(NumberAdditionUI.class.getName()).log(Level.SEVERE, null, ex);
}
// TODO add your handling code here:
}//GEN-LAST:event_jButton1ActionPerformed
/*
* irrelevant code
*/
Update
This is the quick and dirty approach, if you would like me to add a more elegant solution just let me know. Notice that the file names and their relative paths are hard-coded as an array of strings.
Image of the folder hierarchy:
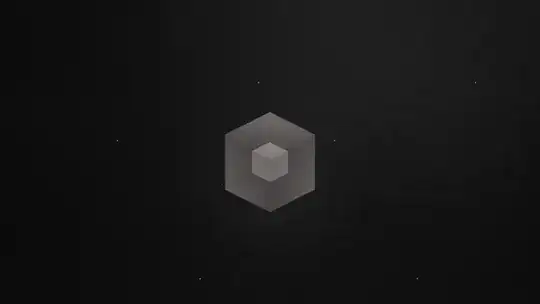
Code:
Note - This will only work on Windows.
import java.io.File;
import java.io.IOException;
public class Driver {
public static void main(String[] args) {
final String[] FILE_NAMES = {"\\files\\READTHIS.txt",
"\\files\\sub-files\\Help.txt",
"\\files\\sub-files\\Config.txt"
};
Runtime rt = Runtime.getRuntime();
// get the absolute path of the directory
File cwd = new File(new File("").getAbsolutePath());
// iterate over the hard-coded file names opening each in notepad
for(String file : FILE_NAMES) {
try {
Process p = rt.exec("notepad " + cwd.getAbsolutePath() + file);
} catch (IOException ex) {
// Logger.getLogger(NumberAdditionUI.class.getName())
// .log(Level.SEVERE, null, ex);
}
}
}
}
Alternative Approach
You could use the
javax.swing.JFileChooser
class to open a dialog that allows the user to select the location of the file they would like to open in Notepad.
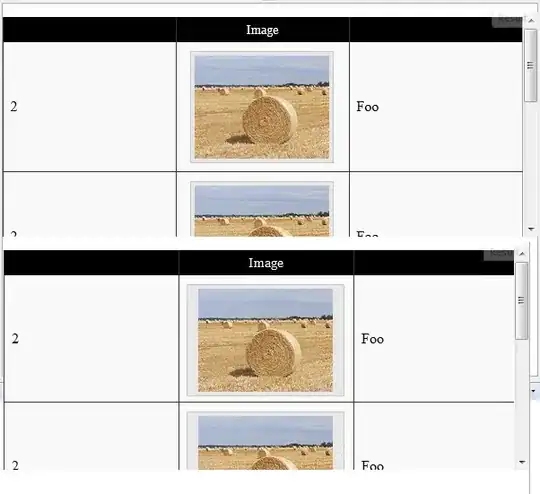
I just coded this quick example using the relevant pieces from your code:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.SwingUtilities;
public class Driver extends JFrame implements ActionListener {
JFileChooser fileChooser; // the file chooser
JButton openButton; // button used to open the file chooser
File file; // used to get the absolute path of the file
public Driver() {
this.fileChooser = new JFileChooser();
this.openButton = new JButton("Open");
this.openButton.addActionListener(this);
// add openButton to the JFrame
this.add(openButton);
// pack and display the JFrame
this.pack();
this.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
// handle open button action.
if (e.getSource() == openButton) {
int returnVal = fileChooser.showOpenDialog(Driver.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
// from your code
Runtime rt = Runtime.getRuntime();
try {
File file = fileChooser.getSelectedFile();
String fileAbsPath = file.getAbsolutePath();
Process p = rt.exec("notepad " + fileAbsPath);
} catch (IOException ex) {
// Logger.getLogger(NumberAdditionUI.class.getName())
// .log(Level.SEVERE, null, ex);
}
} else {
System.exit(1);
}
}
}
public static void main(String args[]) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
Driver driver = new Driver();
}
});
}
}
I've also included a link to some helpful information about the FileChooser
API, provided by Oracle: How to use File Choosers. If you need any help figuring out the code just let me know, via a comment, and I'll try my best to help.
As for including READTHIS.txt
inside the actual java package, take a gander at these other StackOverflow questions: