You have to use customized TabelCellRenderer
with JList
view. Following code should work.
public class VectorTableCellRenderer extends JList<String> implements TableCellRenderer {
@Override
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
if (value instanceof Vector) {
setListData((Vector) value);
}
if (isSelected) {
setBackground(UIManager.getColor("Table.selectionBackground"));
} else {
setBackground(UIManager.getColor("Table.background"));
}
return this;
}
}
EDIT: How to use VectorTableCellRenderer
VectorTableCellRenderer renderer = new VectorTableCellRenderer();
//set TableCellRenderer into a specified JTable column class
table.setDefaultRenderer(Vector.class, renderer);
//or, set TableCellRenderer into a specified JTable column
table.getColumnModel().getColumn(columnIndex).setCellRenderer(renderer);
This is my tested output.
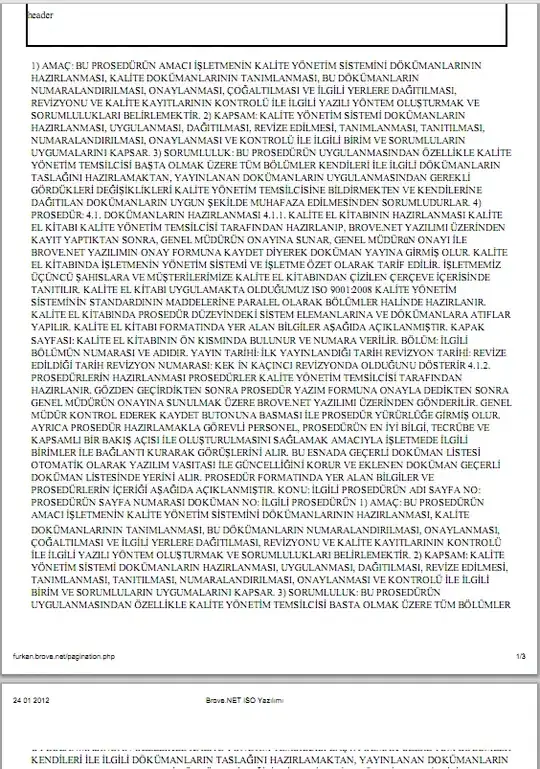