Let's start from the obj format:
this is an example of a simple cube with 3 different textures applied to the opposite faces:
# OBJ File Generated by Meshlab
# Vertices: 8
# Faces: 12
mtllib ./tex_cube.obj.mtl
v -50.00 -50.00 50.00
v -50.00 50.00 50.00
v 50.00 -50.00 50.00
v 50.00 50.00 50.00
v 50.00 -50.00 -50.00
v 50.00 50.00 -50.00
v -50.00 -50.00 -50.00
v -50.00 50.00 -50.00
Pay attention to the path of the mtl file, most of the issues are because the file is not found, and also some 3d tools don't allow relative paths for the mtl file, or for the textures. I suggest you, keep it just side by side with the obj file.
There are 12 triangles, because each side of the cube has been triangulated.
The vt (vertex texture) tag defines how the textures are mapped, in this case by UV (trivially for each face):
vt 1.00 1.00
vt 0.00 1.00
vt 0.00 0.00
vt 1.00 0.00
JSC3D will read the vt tag, the f (faces) tag and the usemtl tag to build the 3d object:
usemtl material_0
f 4/1 2/2 1/3
f 3/4 4/1 1/3
f 8/1 6/2 5/3
f 7/4 8/1 5/3
usemtl material_1
f 6/1 4/2 3/3
f 5/4 6/1 3/3
f 2/1 8/2 7/3
f 1/4 2/1 7/3
usemtl material_2
f 6/1 8/2 2/3
f 4/4 6/1 2/3
f 3/1 1/2 7/3
f 5/4 3/1 7/3
Materials are remapped to the corresponding png files in the mtl file:
newmtl material_0
Ka 0.200000 0.200000 0.200000
Kd 1.000000 1.000000 1.000000
Ks 1.000000 1.000000 1.000000
map_Kd red_tex.png
... and so on for the other two textures, green and blue.
This file has been obtained from following export settings in MeshLab, just to point out that you don't need to export normals, JSC3D will recreate them on the fly:

Sorry for the boring explanation of the obj test file.
If you load this cube in the JSC3D viewer and check the viewer.scene.children[] you will find 3 meshes, because JSC3D has been grouped the 12 triangles of the cube into 3 separate parts, one for each usemtl tag which has been found inside the obj file.
Now, you can get a reference of one of this parts through the PickInfo structure by selecting a face inside the viewer (by clicking with the mouse or by touch), or by code.
For example, if you need to replace the blue texture with another :
function replaceBlueTexture() {
objParts = []; // you can also replace more than one part
objParts[0] = viewer.scene.children[2];
objLoader.setupTexture(objParts, "models/obj/aluminum.png");
}
The result shows like this (left right after loading, right after texture change):

Now, the top and the bottom faces of this cube are aluminum-textured.
EDIT:
The replaceBlueTexture function assume that you already know which mesh shall be replaced.
What if you have to replace the material (or texture) of a mesh by its name?
The obj file is loaded and parsed first, the mtl file is loaded after the obj file, but the material names are already captured and stored by jsc3d in the Mesh object during parsing of the obj file.
If you look at the viewer.scene object, you will see the meshes described above and the associated materials and textures:
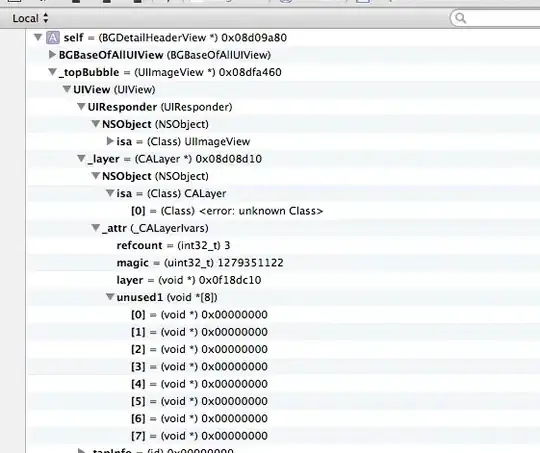
What you need is just only to check the mesh.mtl (or maybe the mesh.material.name - one of both shall be filled in the latest version of jsc3d):
function replaceBlueTextureByName() {
var scene = viewer.getScene();
var meshes = scene.getChildren();
for (var i=0, l=meshes.length; i<l; i++) {
var mesh = meshes[i];
var mat = mesh.material;
if (mat.name == 'mat_blue_tex') {
var objParts = [];
objParts[0] = mesh;
loader.setupTexture(objParts, "models/obj/aluminum.png");
}
}
}
BTW, be sure that the render mode can show textures:
viewer.setParameter('RenderMode', 'texturesmooth');