If your method is declared in some other class like:
class TextColorClass {
func mainAppColor() -> UIColor {
return UIColor(red: 0.2, green: 0.3, blue: 0.4, alpha: 1)
}
}
Then you can use it by make instance of that class this way:
let classInstance = TextColorClass()
yourLBL.textColor = classInstance.mainAppColor()
If it is declared like:
class TextColorClass {
let mainAppColor = UIColor(red: 0.2, green: 0.3, blue: 0.4, alpha: 1)
}
Then you can use it like:
let classInstance = TextColorClass()
yourLBL.textColor = classInstance.mainAppColor
If that method is declare in same class suppose in ViewController
class then you can use it this way:
override func viewDidLoad() {
super.viewDidLoad()
yourLBL.textColor = mainAppColor()
}
func mainAppColor() -> UIColor {
return UIColor(red: 0.2, green: 0.3, blue: 0.4, alpha: 1)
}
UPDATE:
If you want to set any property from storyboard you can use @IBInspectable
as shown in below example code:
import UIKit
@IBDesignable
class PushButtonView: UIButton {
@IBInspectable var fillColor: UIColor = UIColor.greenColor()
@IBInspectable var isAddButton: Bool = true
override func drawRect(rect: CGRect) {
}
}
And assign this class to your button.like shown into below Image:
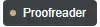
And if you go to the Attribute Inspector you can see custom property for that button.
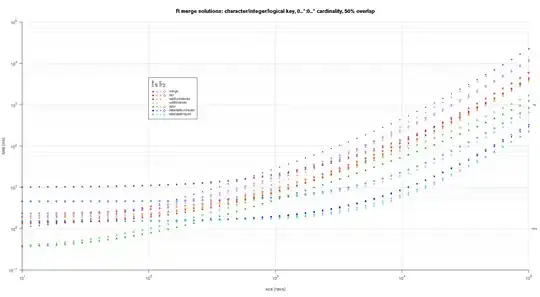
Now you can set it as per your need.
For more Info refer this tutorial:
http://www.raywenderlich.com/90690/modern-core-graphics-with-swift-part-1