matplotlib
doesn't work directly with pixels but rather a figure size (in inches) and a resolution (dots per inch, dpi
)
So, you need to explicitly give a figure size and dpi. For example, you could set your figure size to 1x1 inches, then set the dpi to 60, to get a 60x60 pixel image.
You also have to remove the whitespace around the plot area, which you can do with subplots_adjust
import numpy as np
import matplotlib.pyplot as plt
plt.figure(figsize=(1,1))
A = np.random.rand(60,60,3)
plt.imshow(A,
cmap=plt.cm.gist_yarg,
interpolation='nearest',
aspect='equal',
shape=A.shape)
plt.subplots_adjust(left=0,right=1,bottom=0,top=1)
plt.savefig('myfig.png',dpi=60)
That creates this figure:
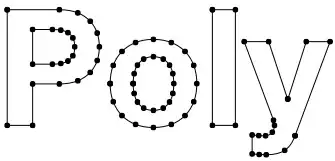
Which has a size of 60x60 pixels:
$ identify myfig.png
myfig.png PNG 60x60 60x60+0+0 8-bit sRGB 8.51KB 0.000u 0:00.000
You might also refer to this answer, which has lots of good information about figure sizes and resolutions.