There is no way to track down redirects in a general way - you have to trigger an event right before the redirect is processed, so you have to know how this happen.
Why ? Because a JS redirection is processed by the browser and not on the server side - which means the only way to trigger an SQL insert is to know how the redirection is triggered. This is basically what you want to achieve:
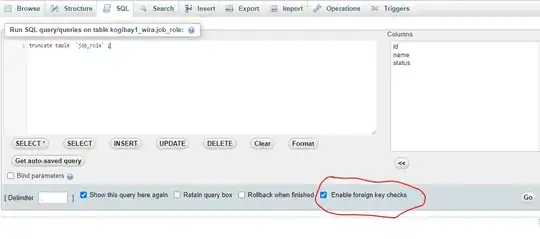
What you can do to locate the redirection
- Search for every occurences of
window.location
in your JS files - you could search for the URL that you've been redirected too (all your local js files and the external ones you include)
- Disable (with comments) the external
<iframe>
you're using. An iframe can do a window.top.location.href
to redirect the main page.
- An other way to redirect the page would be to trigger a
click
event on a link through JS. I guess by searching for the redirect URL in your js file you could locate that too.
- Last, if the redirection happens before the page is load, it could a PHP redirection - looking for
header
calls that redirect to the URL in your php files would catch that.
What can be done when the redirect is found
- It's a JS redirection (
window.location
)
If it's inside a local file, you can just comment it if you want to disable it, if you want to keep it but track it, add this code right before the window.location
call (it's jQuery, it will make an AJAX request to /log-redirects.php
) :
$.post( "/log-redirects.php", function( data ) {
// ADD the window.location line here
});
It's really important that you move the window.location
call inside the AJAX callback, so the redirection will be made only when the ajax call is finished.
If the redirection happens in an external js, you could not probably do anything besides removing the whole JS file - maybe get the JS file content, remove the redirection and include it locally.
One thing you could try is to add a listener on the beforeunload
event - though you'll have to do the correct check on the event object to see if it's the redirection you're looking for, as it'll catch any unload events.
window.addEventListener("beforeunload", function (e) {
// do something
return false; // will result to a prompt to the user asking him if he really want to redirect
// return this only for the redirect you're looking for
});
Please note that this method doesn't seems to be completely reliable.
- It's from an
<iframe>
You can add the sandbox
attribute to the iframe to prevent it to do any redirect. Please note that this attribute is not supported in IE9 and lower.
<iframe src="http://youriframeurl.com/" sandbox=""></iframe>
- It's a click event triggered through JS
You can do the same thing as for the first point. You just want to add a listener to the link (using on
if it's generated after DOM is load) :
$('a#mylink').on('click', function(e) {
// do something
e.preventDefault(); // add this if you want to cancel the redirection
});
- It's a
header
redirection (PHP)
That's an easy case - just add the code you want before the header
call.
Conclusion
I suggest to work first with the beforeunload
event to track the redirection - doing a console.log(event);
could give you some insights on the cause of the redirection.
Good luck!