JUnit has a rule called ErrorCollector that can be used to gather progressive stats about a test and report at the end of the execution.
Don't combine Assert and ErrorCollector, you will lose ErrorCollector information if your test fails from an Assert
At the end of your test any failures will be gathered and listed collectively in the JUnit output.
package stackoverflow.proof.junit;
import org.hamcrest.core.Is;
import org.hamcrest.core.IsEqual;
import org.hamcrest.core.IsNull;
import org.junit.Assert;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ErrorCollector;
/**
* Test case for demonstrating some Junit Rule behaviors.
* <p/>
* Requires at least JUnit 4.7
*
* @see "http://stackoverflow.com/questions/33183789/not-able-to-continue-when-an-assert-condition-fails-in-selenium-webdriver/33183882#33183882"
* @author http://stackoverflow.com/users/5407189/jeremiah
* @since Oct 17, 2015
*
*/
public class JUnitRulesDemoTest {
/**
* Collector which can be used to track problems through an event series and still allow a test to run to
* completion.
*/
@Rule
public ErrorCollector collector = new ErrorCollector();
/**
* Test illustrating ErrorCollector behavior.
*
*/
@Test
public void testErrorCollector() {
collector.checkThat("These things don't match", new Object(), IsEqual.equalTo(new Object()));
collector.checkThat(new Object(), IsNull.notNullValue());
try {
throw new Exception("Demonstration Exception in collector");
} catch (Exception ex) {
collector.addError(ex);
}
collector.checkThat("Status does not match!", true, Is.is(true));
System.out.println("Done.");
}
/**
* Test illustrating ErrorCollector behavior when an Assert Fails the Test.
*
*/
@Test
public void testErrorCollectorWithAssert() {
collector.checkThat("These things don't match", new Object(), IsEqual.equalTo(new Object()));
collector.checkThat(new Object(), IsNull.notNullValue());
try {
throw new Exception("Demonstration Exception in collector");
} catch (Exception ex) {
collector.addError(ex);
}
//Test stops here. You won't see "done", nor will you see any of the previous ErrorCollector status'
Assert.assertTrue(false);
collector.checkThat("Status does not match!", true, Is.is(true));
System.out.println("Done.");
}
}
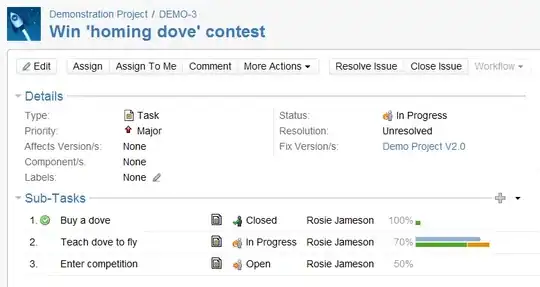
A Rule must:
- Be annotated with @Rule
- Be declared public in your test.
Proposed Solution:
In the case you list above you should remove the try/catch block and use the Is Matcher instead of org.junit.Assert.assertEquals:
@Rule
public ErrorCollector collector = new ErrorCollector();
@Test
public void testSomething() {
boolean status = doStatusEval();
collector.checkThat("Disabled user can register for workshop", false, Is.is(status));
//Continue adding things to the collector.
}
Best of Luck!