There should be some much more elegant solution, but here we go:
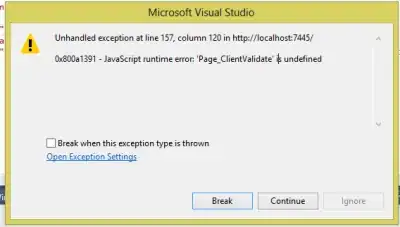
List<Point> reducePlotLines(List<Point> points)
{
float limit = 0.1f;
List<Point> newPoints = new List<Point>();
Dictionary<int, float> slopes = new Dictionary<int, float>();
List<int> toRemove = new List<int>();
for (int i = 1; i < points.Count; i++)
{
if ( points[i-1].Y - points[i].Y == 0) slopes.Add(i, float.MaxValue);
else slopes.Add(i,
1f * (points[i-1].X - points[i].X) / (points[i-1].Y - points[i].Y));
}
newPoints.Add(points[0]);
for (int i = slopes.Count - 2; i > 0; i--)
{
if (Math.Abs(slopes[i] - slopes[i + 1]) < limit)
toRemove.Add(i);
}
foreach (int i in toRemove) slopes.Remove(i);
newPoints.Add(points[points.Count-1]);
foreach (KeyValuePair<int, float> kv in slopes) newPoints.Add(points[kv.Key]);
return newPoints;
}
The above chart was created like this:
private void button7_Click(object sender, EventArgs e)
{
chart1.Series.Clear(); chart1.Series.Add("S1");
chart1.Series.Add("S2"); chart1.Series.Add("S3");
List<Point> points = new List<Point>();
points.Add(new Point(1,2)); points.Add(new Point(2,5));
points.Add(new Point(3,8)); points.Add(new Point(4,6));
points.Add(new Point(5,4)); points.Add(new Point(6,2));
points.Add(new Point(7,4));
chart1.Series[0].ChartType = SeriesChartType.Line;
chart1.Series[1].ChartType = SeriesChartType.Point;
chart1.Series[2].ChartType = SeriesChartType.Point;
chart1.Series[0].Points.Clear();
foreach (Point pt in points)
{
chart1.Series[0].Points.AddXY(pt.X, pt.Y);
chart1.Series[1].Points.AddXY(pt.X, pt.Y);
}
List<Point> reducedPoints = reducePlotLines(points);
foreach (Point pt in reducedPoints)
{
chart1.Series[2].Points.AddXY(pt.X, pt.Y);
}
}
Note that I had to change your data as it contained only one interpolated point.