FYI Going forward, if you continue to use StackOverflow, you should be sure to include the tags for WinForms, WPF, or ASP.NET (or at least specify it in your post) and make sure you state the full type of the control you're using. You use a DataGridView
but you named it GridView01
, which causes confusion for us because there is the WinForms DataGridView
control and the ASP.NET GridView
control which have different properties and methods associated with them. So one person's suggested answer might not work because they mistook your control for a different one. The other StackOverflow question I linked in my comment on your original post is for an ASP.NET GridView
control, which is why we thought you should be accessing the Text
property. Only the error message which states it's actually a DataGridView
was I able to determine which control you're actually using ;)
Anyways, I created a small little WinForms application with just a DataGridView
and Textbox
control on it. In my Form_Load
event I added some rows with text data to my DataGridView
like so:
private void Form1_Load(object sender, EventArgs e)
{
for (int i = 0; i < 10; i++)
{
DataGridViewRow dgvr = (DataGridViewRow)dataGridView1.Rows[0].Clone();
for (int j = 0; j < 3; j++)
{
switch j)
{
case 0:
dgvr.Cells[j].Value = "A - " + i;
break;
case 1:
dgvr.Cells[j].Value = "B - " + i;
break;
case 2:
dgvr.Cells[j].Value = "C - " + i;
break;
}
}
dataGridView1.Rows.Add(dgvr);
}
}
You of course don't need to do that, I'm just showing you what I did so there are no mysteries.
Then I added a SelectionChanged
event to my DataGridView
control. I don't seem to have a Mouse_Click
event available, so instead I set myDataGridView.SelectionMode = FullRowSelect
in the properties sheet. This makes it so that you can't select a single cell, but rather clicking a cell selects the entire row. This guarantees* there'll be something in the SelectedRows
property.
private void dataGridView1_SelectionChanged(object sender, EventArgs e)
{
if (dataGridView1.SelectedRows.Count > 0)
{
textBox1.Text = dataGridView1.SelectedRows[0].Cells[0].Value.ToString();
}
}
And this is properly dumping the value of the first cell within the selected row into my textbox:
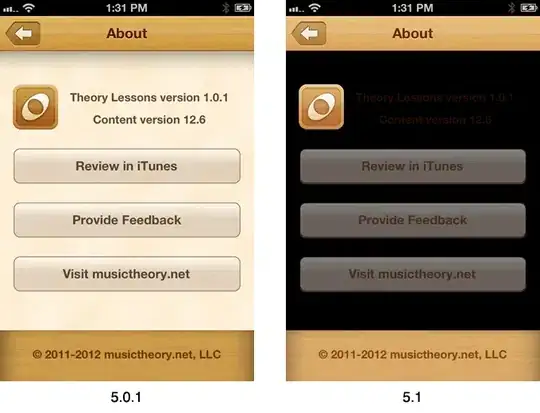
Then all you need to do is set which textbox uses which cell index.
Edit: Sample 'Immediate Window' output;

Edit 2: Make sure the application is running, set the breakpoint, select a new row in your DataGridView
and then enter that line in the immediate window like shown here: https://i.stack.imgur.com/gR8HM.png