Here's an approach that works quite well for spoofing the PluginArray (notice the Object.setPrototypeOf
):
(function generatePluginArray() {
const pluginData = [
{ name: "Chrome PDF Plugin", filename: "internal-pdf-viewer", description: "Portable Document Format" },
{ name: "Chrome PDF Viewer", filename: "mhjfbmdgcfjbbpaeojofohoefgiehjai", description: "" },
{ name: "Native Client", filename: "internal-nacl-plugin", description: "" },
]
const pluginArray = []
pluginData.forEach(p => {
function FakePlugin () { return p }
const plugin = new FakePlugin()
Object.setPrototypeOf(plugin, Plugin.prototype);
pluginArray.push(plugin)
})
Object.setPrototypeOf(pluginArray, PluginArray.prototype);
return pluginArray
})()
Console output:
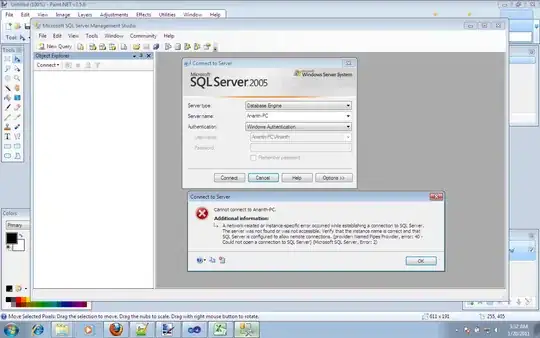
I haven't added the MimeType property yet, but that should be accomplishable in a similar manner.
Feel free to submit a PR in case you flesh this out (I've developed a plugin for puppeteer that's implementing various detection evasion techniques):
https://github.com/berstend/puppeteer-extra/tree/master/packages/puppeteer-extra-plugin-stealth
Edit: I've had some spare time and added code to fully emulate navigator.plugins
and navigator.mimeTypes
here. It even mocks functional methods, instance types and .toString properties, to make them look native and resemble a normal Google Chrome.