The java.util
date-time API and their formatting API, SimpleDateFormat
are outdated and error-prone. It is recommended to stop using them completely and switch to the modern date-time API* .
Since your date-time string has timezone information, parse it into ZonedDateTime
which you can convert to other java.time types and if required, format into the desired formats.
Demo:
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.OffsetDateTime;
import java.time.ZoneOffset;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeFormatterBuilder;
import java.time.format.TextStyle;
import java.util.Locale;
public class Main {
public static void main(String[] args) {
String strDateTime = "Mon Nov 09 2015 00:00:00 GMT+0530 (India Standard Time)";
DateTimeFormatter dtfInput = new DateTimeFormatterBuilder()
.parseCaseInsensitive()
.appendPattern("E MMM d uuuu H:m:s")
.appendLiteral(" ")
.appendZoneId()
.appendPattern("X")
.appendLiteral(" ")
.appendLiteral("(")
.appendGenericZoneText(TextStyle.FULL)
.appendLiteral(')')
.toFormatter(Locale.ENGLISH);
ZonedDateTime zdt = ZonedDateTime.parse(strDateTime, dtfInput);
OffsetDateTime odt = zdt.toOffsetDateTime();
System.out.println(odt);
// To LocalDate, LocalDateTime etc.
LocalDate localDate = odt.toLocalDate();
LocalDateTime localDateTime = odt.toLocalDateTime();
System.out.println(localDate);
System.out.println(localDateTime);
// Get the formatted string
DateTimeFormatter dtfOutput = DateTimeFormatter.ofPattern("dd/MM/uuuu", Locale.ENGLISH);
String formatted = dtfOutput.format(localDate);
System.out.println(formatted);
// In UTC
odt = odt.withOffsetSameInstant(ZoneOffset.UTC);
System.out.println(odt);
}
}
Output:
2015-11-09T00:00+05:30
2015-11-09
2015-11-09T00:00
09/11/2015
2015-11-08T18:30Z
Learn more about the modern date-time API from Trail: Date Time.
Support for OffsetDateTime
in JDBC:
Starting with JDBC 4.2, you can use OffsetDateTime
directly in your code e.g.
PreparedStatement st = conn.prepareStatement("INSERT INTO mytable (columnfoo) VALUES (?)");
st.setObject(1, odt);
st.executeUpdate();
st.close();
The support for java.time API in JDBC Java SE 8 (JDBC 4.2) came without requiring any public JDBC API changes. The setObject
and getObject
methods support java.time types as per the following mapping:
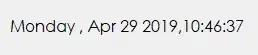
Note for PostgreSQL™: ZonedDateTime
, Instant
and OffsetTime
/ TIME WITH TIME ZONE
are not supported. Also, the OffsetDateTime
instances need to be in UTC (have offset 0).
* For any reason, if you have to stick to Java 6 or Java 7, you can use ThreeTen-Backport which backports most of the java.time functionality to Java 6 & 7. If you are working for an Android project and your Android API level is still not compliant with Java-8, check Java 8+ APIs available through desugaring and How to use ThreeTenABP in Android Project.