You can't prevent that people use the drop-down list, but you can make sure that functionality such as "Reply" and "Delete" isn't shown in that list by encrypting the document using an owner password, making sure that you don't set the option that allows people to add annotations. Once the PDF is encrypted, you'll notice that the entries in the drop-down list are limited. (See How to protect an already existing PDF with a password? to find out how to encrypt a document.)
Changing the size of an annotation is a matter of replacing the PDF rectangle that was defined for that annotation. Please take a look at the MovePopup example.
We have the following annotation and popup:
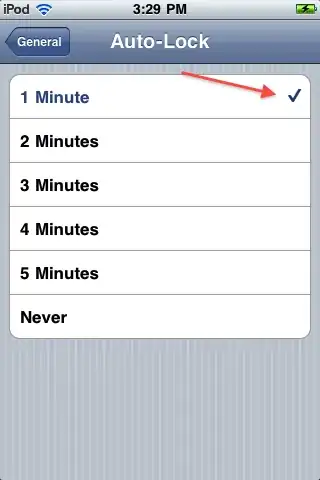
Incidentally, I know that the text annotation is the first annotation in the /Annots
array and that the popup is the second one. This means that I can cut some corners in my code:
public void manipulatePdf(String src, String dest) throws IOException, DocumentException {
PdfReader reader = new PdfReader(src);
PdfDictionary page = reader.getPageN(1);
PdfArray annots = page.getAsArray(PdfName.ANNOTS);
PdfDictionary sticky = annots.getAsDict(0);
PdfArray stickyRect = sticky.getAsArray(PdfName.RECT);
PdfRectangle stickyRectangle = new PdfRectangle(
stickyRect.getAsNumber(0).floatValue() - 120, stickyRect.getAsNumber(1).floatValue() -70,
stickyRect.getAsNumber(2).floatValue(), stickyRect.getAsNumber(3).floatValue() - 30
);
sticky.put(PdfName.RECT, stickyRectangle);
PdfDictionary popup = annots.getAsDict(1);
PdfArray popupRect = popup.getAsArray(PdfName.RECT);
PdfRectangle popupRectangle = new PdfRectangle(
popupRect.getAsNumber(0).floatValue() - 250, popupRect.getAsNumber(1).floatValue(),
popupRect.getAsNumber(2).floatValue(), popupRect.getAsNumber(3).floatValue() - 250
);
popup.put(PdfName.RECT, popupRectangle);
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(dest));
stamper.close();
}
I have made both rectangles bigger by subtracting 120, 70, 250,... user units from the x
and y
values in the /Rect
value of the annotations here and there.
The result looks like this:
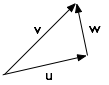
Both the text annotation icon and the actual popup with the text are now bigger.
It is up to you to adapt the code, so that:
- You find the actual popup annotation that you want to enlarge,
- You change the rectangle of that annotation with the amount of user units of your choice.