I managed to get a solution based on this question and the answers therein. It seems that intrafacet interpolation is not built into pyplot
, so you need a workaround by creating a finer interpolating mesh on your data.
Using your example data in MATLAB:
x = [0 1 1; 0 1 0]';
y = [0 0 1; 0 1 1]';
z = [0 1 2; 0 2 3]';
fill(x,y,z);
produces this plot (which will be a control for us):
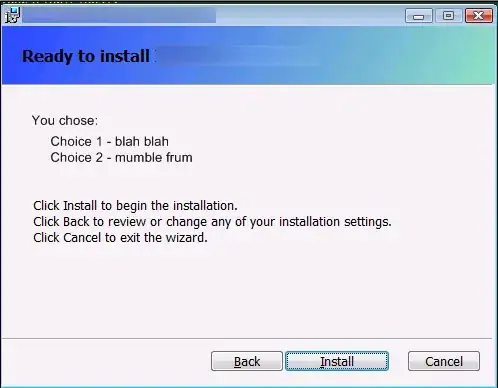
First you have to determine how your data are represented in python
. The natural inputs to the functions I'm going to use are 1d arrays, so you might throw away the multiplicities in your data. If you don't actually care for the triangles, but only the z
landscape, then you can let python
do the triangulation for you:
from matplotlib import tri, cm
import matplotlib.pyplot as plt
x=[0, 1, 1, 0]
y=[0, 0, 1, 1]
z=[0, 1, 2, 3]
triang=tri.Triangulation(x,y) #Delaunay triangulation of input
refiner=tri.UniformTriRefiner(triang)
interp=tri.LinearTriInterpolator(triang,z) #linear interpolator
new,new_z=refiner.refine_field(z,interp,subdiv=6) #refined mesh; uses 4**subdiv!
fig = plt.figure()
plt.tripcolor(new.x,new.y,new_z,cmap=cm.jet)
The result:

You can see that the result is different from the control, because matplotlib
chose another triangulation as we did in MATLAB. If your triangles are small, and you only need a smooth figure, then this should suffice.
However, it is possible to provide the triangulation manually. It would be less then trivial to convert your MATLAB input to a format that is palatable by python
, so it's up to how desperate you are. For our small example I can choose the triangles manually:
x=[0, 1, 1, 0]
y=[0, 0, 1, 1]
z=[0, 1, 2, 3]
tridat=[[0,3,2],[0,2,1]] # 2 triangles in counterclockwise order
triang=tri.Triangulation(x,y,tridat) #this has changed
refiner=tri.UniformTriRefiner(triang)
interp=tri.LinearTriInterpolator(triang,z) #linear interpolator
new,new_z=refiner.refine_field(z,interp,subdiv=6) #refined mesh
fig = plt.figure()
plt.tripcolor(new.x,new.y,new_z,cmap=cm.jet)
Result:
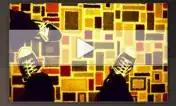
This is clearly comparable to the MATLAB version. For a smoother interpolation you can increase subdiv
in the code, but do so carefully: each triangle is split into 4^subdiv
parts, so the memory need of plotting grows very rapidly with this parameter.