You can use SpannableString and ClickableSpan. this Activity, for example, creates TextView with your text and manages clicks on each word:
public class MainActivity extends AppCompatActivity {
Activity activity;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
activity = this;
TextView textView = (TextView) findViewById(R.id.textView);
String text = "up down Antidisestablishment over took dropped lighten with from throught fell on up down Antidisestablishment over took dropped lighten with from throught fell on";
String[] textArray = text.split(" ");
SpannableString ss = new SpannableString(text);
int start = 0;
int end = 0;
for(final String item : textArray){
end = start + item.length();
ClickableSpan clickableSpan = new ClickableSpan() {
@Override
public void onClick(View textView) {
Toast.makeText(activity, "Say " + item+ "!", Toast.LENGTH_SHORT).show();
}
@Override
public void updateDrawState(TextPaint ds) {
super.updateDrawState(ds);
ds.setUnderlineText(false);
}
};
ss.setSpan(clickableSpan, start, end, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
start += item.length()+1;
}
textView.setText(ss);
textView.setMovementMethod(LinkMovementMethod.getInstance());
textView.setHighlightColor(Color.TRANSPARENT);
}
}
and here is activity_main.xml:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity"
android:padding="5dp">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp"
android:textColor="#000000"
android:textColorLink="#000000"/>
</LinearLayout>
if you click on any word you get popup with this word
EDIT: to justify the text use the library android-justifiedtextview
But not the library from gradle, there are the old version which does not support SpannableString
. I recommend just copy the class JustifyTextView
from git to your project. Then you can use this view in your .xml
like:
<com.yourdomain.yourproject.JustifyTextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp"
android:textColor="#000000"
android:textColorLink="#000000"/>
here is what I got with this library:
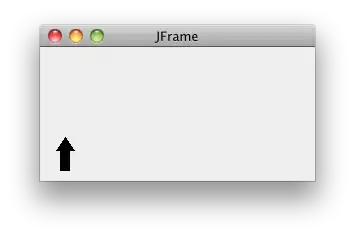
you also can modify the library to keep last line unjustified. Every word in the text is still clickable.