An Object Oriented approach:
The following approach utilizes a JSON config file. The file is parsed, using Google's GSON library, and the information in the configuration file is what the application uses to construct the buttons.
This has been tested and verified to work. You may need to remove or change the application paths for your application to function correctly.
Note: You will need a dependency management system i.e. Maven or Gradle to pull in GSON or you could download a jar and link it manually.
Screenshot
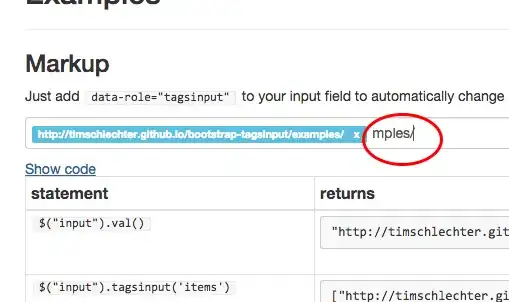
Structure
This is the skeleton structure for the application.
> AppLauncher/
> src/
> app/
> component/
- AppButton.java
> config/
> dto/
- AppConfig.java
- RootConfig.java
> view/
- AppDashboard.java
> resources/
- appConfig.json
- AppLauncher.java
Classes and Files
AppLauncher
This is the application launcher which starts the application after reading the config file.
import javax.swing.JFrame;
import javax.swing.SwingUtilities;
import app.config.dto.RootConfig;
import app.config.reader.ConfigReader;
import app.view.AppDashboard;
public class AppLauncher {
public static final String APP_TITLE = "App Launcher";
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
JFrame frame = new JFrame(APP_TITLE);
RootConfig config = ConfigReader.read("/resources/appConfig.json");
AppDashboard dashboard = new AppDashboard(config);
System.out.println(config);
frame.setContentPane(dashboard);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
});
}
}
AppDashboard
This view holds the buttons for each of the applications defined in the config.
package app.view;
import java.awt.FlowLayout;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JPanel;
import app.component.AppButton;
import app.config.dto.AppConfig;
import app.config.dto.RootConfig;
public class AppDashboard extends JPanel {
private static final long serialVersionUID = -3885872923055669204L;
private RootConfig config;
private List<AppButton> buttons;
public AppDashboard(RootConfig config) {
super(new FlowLayout());
this.config = config;
buttons = new ArrayList<AppButton>();
for (AppConfig appConfig : config.getApplications()) {
buttons.add(new AppButton(appConfig));
}
createChildren();
}
protected void createChildren() {
for (AppButton button : buttons) {
this.add(button);
}
}
}
AppButton
This is the application button which has a text label and a launch action when the button is triggered. This can be extended to render the icon of the application.
package app.component;
import java.awt.event.ActionEvent;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.List;
import javax.swing.AbstractAction;
import javax.swing.JButton;
import app.config.dto.AppConfig;
public class AppButton extends JButton {
private static final long serialVersionUID = -730609116042003884L;
private AppConfig config;
public AppButton(AppConfig config) {
super();
this.config = config;
setAction(new AbstractAction(config.getName()) {
private static final long serialVersionUID = -2999563123610952893L;
@Override
public void actionPerformed(ActionEvent e) {
try {
launch();
} catch (IOException e1) {
e1.printStackTrace();
}
}
});
}
private String[] procArguments() {
List<String> commands = config.getArguments();
commands.add(0, config.getPath());
return commands.toArray(new String[commands.size()]);
}
/**
* @see http://stackoverflow.com/questions/13991007/execute-external-program-in-java
*/
public void launch() throws IOException {
String[] args = procArguments();
Process process = new ProcessBuilder(args).start();
InputStream is = process.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
BufferedReader br = new BufferedReader(isr);
String line;
System.out.printf("Output of running %s is:", Arrays.toString(args));
while ((line = br.readLine()) != null) {
System.out.println(line);
}
}
}
ConfigReader
This handles reading the JSON config file and marshaling it into a java DTO (data transfer object) which will be used by the application's components.
package app.config.reader;
import java.io.InputStreamReader;
import com.google.gson.Gson;
import app.config.dto.RootConfig;
public class ConfigReader {
private static ClassLoader loader = ConfigReader.class.getClassLoader();
public static RootConfig read(String filename) {
return new Gson().fromJson(getInputStream(filename), RootConfig.class);
}
private static InputStreamReader getInputStream(String filename) {
return new InputStreamReader(loader.getResourceAsStream(filename));
}
}
RootConfig
This it the root of the config which holds the application configurations.
package app.config.dto;
import java.util.List;
public class RootConfig {
private List<AppConfig> applications;
public List<AppConfig> getApplications() {
return applications;
}
public void setApplications(List<AppConfig> applications) {
this.applications = applications;
}
@Override
public String toString() {
return "RootConfig [applications=" + applications + "]";
}
}
AppConfig
This is an application configuration describing the application.
package app.config.dto;
import java.util.List;
public class AppConfig {
private String name;
private String path;
private List<String> arguments;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public List<String> getArguments() {
return arguments;
}
public void setArguments(List<String> arguments) {
this.arguments = arguments;
}
@Override
public String toString() {
return "AppConfig [name=" + name + ", path=" + path + ", arguments=" + arguments + "]";
}
}
appConfig.json
This is the configuration file which will be read by the application to create the buttons.
{
"applications" : [
{
"name" : "Chrome",
"path" : "C:/Program Files (x86)/Google/Chrome/Application/chrome.exe",
"arguments" : []
}, {
"name" : "Eclipse",
"path" : "C:/Eclipse/current/eclipse.exe",
"arguments" : []
}, {
"name" : "Control Panel",
"path" : "C:/Windows/System32/control.exe",
"arguments" : []
}
]
}
pom.xml Dependencies
These are Maven dependencies which are required for the program to parse the JSON file. You may use another parsing library such as Jackson or JsonLib.
<dependencies>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.4</version>
</dependency>
</dependencies>