To use transparency, you need to work with alpha
channel, in BGRA color space. alpha = 0
means fully transparent, while alpha = 255
means opaque colors.
You need to create a CV_8UC4
image (aka Mat4b
), or convert a 3 channel image to 4 channel with cvtColor(src, dst, COLOR_BGR2BGRA)
, and draw a transparent filled shape, with alpha channel equals to 0.
If you load a 4 channel image, remember to use imread("path_to_image", IMREAD_UNCHANGED);
Transparent triangle (the transparency is rendered as white here, you can see that the image is in fact transparent opening it with your preferred image viewer):
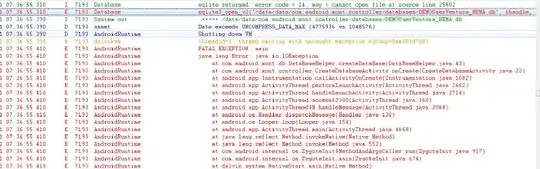
Code:
#include <opencv2/opencv.hpp>
#include <vector>
using namespace std;
using namespace cv;
int main()
{
// Create a BGRA green image
Mat4b image(300, 300, Vec4b(0,255,0,255));
vector<Point> vertices{Point(100, 100), Point(200, 200), Point(200, 100)};
vector<vector<Point>> pts{vertices};
fillPoly(image, pts, Scalar(0,0,0,0));
// ^ this is the alpha channel
imwrite("alpha.png", image);
return 0;
}
NOTE
imshow
won't show transparency correctly, since it just ignores the alpha
channel.
C++98 version
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// Create a BGRA green image
Mat4b image(300, 300, Vec4b(0, 255, 0, 255));
Point vertices[3];
vertices[0] = Point(100, 100);
vertices[1] = Point(200, 200);
vertices[2] = Point(200, 100);
const Point* ppt[1] = { &vertices[0] };
int npt[] = { 3 };
fillPoly(image, ppt, npt, 1, Scalar(0, 0, 0, 0), 8);
imwrite("alpha.png", image);
imshow("a", image);
waitKey();
return 0;
}