The best way to create games like this is to use something like OpenGL, DirectX, XNA etc, but you can also use GDI+ and Graphics.DrawImage
.
But one thing you should know is that pretty much nothing's impossible when it comes to programming. :)
This is a solution I use for pictureboxes with proper transparent background. Just keep in mind that moving the picturebox over other controls/pictureboxes may cause it to lag, as it has to recursively redraw everything behind it:
1) First, create a custom component (found in the "Add New Item" menu in VS/VB).
2) Give it a name of your choice (ex: TransparentPictureBox
).
3) Make it inherit from the original PictureBox
.
Public Class TransparentPictureBox
Inherits PictureBox
End Class
4) Paste the following code inside the class:
Protected Overrides Sub OnPaintBackground(e As System.Windows.Forms.PaintEventArgs)
MyBase.OnPaintBackground(e)
If Parent IsNot Nothing Then
Dim index As Integer = Parent.Controls.GetChildIndex(Me)
For i As Integer = Parent.Controls.Count - 1 To index + 1 Step -1
Dim c As Control = Parent.Controls(i)
If c.Bounds.IntersectsWith(Bounds) AndAlso c.Visible = True Then
Dim bmp As New Bitmap(c.Width, c.Height, e.Graphics)
c.DrawToBitmap(bmp, c.ClientRectangle)
e.Graphics.TranslateTransform(c.Left - Left, c.Top - Top)
e.Graphics.DrawImageUnscaled(bmp, Point.Empty)
e.Graphics.TranslateTransform(Left - c.Left, Top - c.Top)
bmp.Dispose()
End If
Next
End If
End Sub
This code overrides the PictureBox's OnPaintBackground
event, thus drawing it's own background by drawing every control behind it onto the background.
5) Build your project (see pictures below if you don't know how).
6) Select your component from the ToolBox and add it to your form.
Hope this helps!
Building your project
Open the Build
menu in Visual Basic and press Build <your project name here>
.
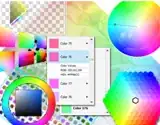
Add your component from the ToolBox
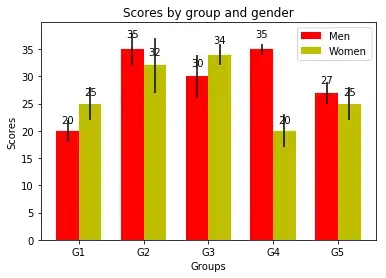