Another simple way to count how many white spots there are in the image is to first convert your image to black and white, remove the white border, then count how many there are remaining. Use bwlabel
and determine the value seen in the second output variable. To clear the white border, use imclearborder
. BTW, this assumes you have the Image Processing Toolbox:
%// Read image directly from StackOverflow
im = im2bw(imread('https://i.stack.imgur.com/9hU5O.png'));
%// Remove white border surrounding image
im = imclearborder(im);
%// Count how many white spots there are
[~,num] = bwlabel(im);
I get:
>> num
num =
18
Now in the case of your real example, you have some noisy pixels. There are several ways you can get rid of those isolated streaks. The thing that worked for me was to use morphological opening with a 5 x 5 square structuring element. Once you do this, this removes any noisy pixels that have an area of less than 5 x 5, and leaves any shape whose area is larger than 5 x 5 shape alone. Use a combination of imopen
and strel
to help you do this:
%// Read in the new image and convert to black and white
im = im2bw(imread('https://i.stack.imgur.com/HCvAa.png'));
%// Clear the border
im = imclearborder(im);
%// Define the structuring element
se = strel('square', 5);
%// Open the image
out = imopen(im, se);
%// Now count the objects
[~,num] = bwlabel(out);
I get:
>> num
num =
18
Also, if you're curious, this is what the cleaned up image looks like:
>> imshow(out);
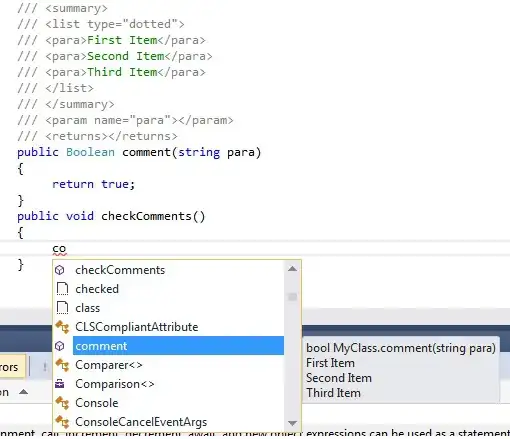
This processing was done on the new image you posted, and you can see that the noisy white pixels have been removed due to the opening operation.