[update] Using new Integer(int)
is guaranteed to always result in a new object whereas Integer.valueOf(int)
allows caching of values to be done by the compiler, class library, or JVM.
To explain I have written below code and used javap
tool, command to generate below code I used javap -c <Classname>
which gives you byte code.
Integer x = new Integer(0), y;
y=x;
x+=0;
System.out.println(x==y); // prints false

If you will see in the above code it creates new object with dynamic memory allocator which is new
. Now in second case as below:
Integer x = 0, y;
y=x;
x+=0;
System.out.println(x==y); // prints true
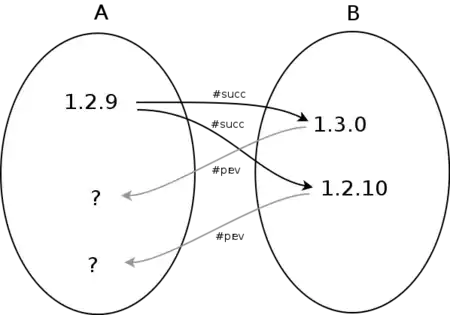
As said by Peter it uses valueOf
method which means it is comparing same object at run time so it will return true
with object comparison operator (==
). But in first case it was creating new object which is conspicuous in below debugging snapshot:
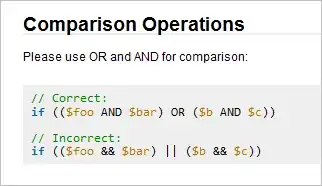
I hope this helps. :)
By the way Kevin Esche answer also adds to the question. Because it is basically referencing to cached object, try to relate it in case of String
. If you are using new String("some_string")
it will create new object else if available it will use from string pool
. And remember you are using wrapper classes not primitive.