I would like to provide a detailed answer in order for you not only understand the Logical (AND) and (OR) but, also how the operators in general are evaluated and what are their order of precedence. There are many ways to evaluate multiple logical expressions.
The first step is to understand the truth table for OR and AND shown below (Source):
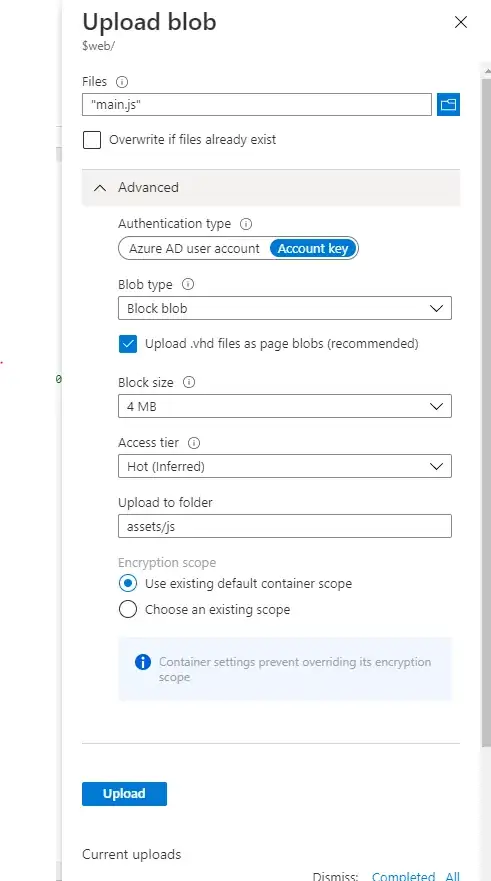
As shown above, for different values of x and y the X AND Y (X && Y) and X OR Y (X || Y) yield different values. If you pay attention below AND operation requires both X and Y to be true in order for the result to be true however in case of OR if either X or Y is true then the result is true.
You need to know the above table for AND and OR by heart.
If you wish to be able to evaluate multiple logical expressions (ANDs and ORs), De Morgen's law can help you how to do it using NOT operation.
The rules can be expressed in English as:
The negation of a conjunction is the disjunction of the negations. The
negation of a disjunction is the conjunction of the negations.
or informally as:
"not (A and B)" is the same as "(not A) or (not B)"
also,
"not (A or B)" is the same as "(not A) and (not B)".
Read more on De Morgen's law and how to evaluate multiple logical expressions using it in here and here.
Precedence of logical AND and OR are left to right. See below table (Source) and that should give you an idea which operators are right to left and which ones are left to right as well as order of their precedence for example () and []
has the highest precedence and assignments have the lowest precedence among operators. If you notice
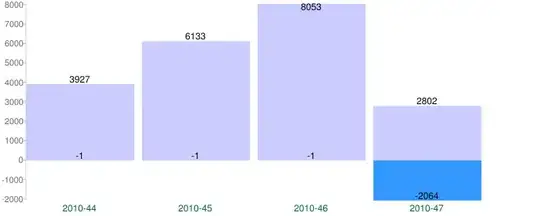
back to your example
if ( a || b || c || d)
According to truth table there are 16 possible combinations starting from
a=true,b=true,c=true,d=true
...
...
...
a=false,b=false,c=false,d=false
As long as any of the a,b,c,d are true then outcome is true hence, out of 16 possible combinations 15 will be true and last one will be false because all a,b,c,d are false.
ON whether below is same as above? Yes.
if ((a || b) || (c || d))
The only difference between the 2 is that in the second one the brackets are evaluated first hence (a || b) is evaluated then ORd with evaluation result of (c || d) but. The same logic true for above is true here too. As long as one of a,b,c,d are true then outcome is true.
In regards to below, yes the two evaluate to same outcome.
if ( a && b && c && d)
vs
if ((a && b) && (c && d))
Same as above 16 possible combinations for different values for a,b,c,d. The only true outcome for the above is when all a,b,c,d are true. Hence only one true and another 15 false.