If you choose Python2.7 in your project. Counter()
can be used in this case:
Python 2.7.16 (default, Jun 5 2020, 22:59:21)
[GCC 4.2.1 Compatible Apple LLVM 11.0.3 (clang-1103.0.29.20) (-macos10.15-objc- on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> x={1:['a','b','c']}
>>> y={1:['d','e','f'],2:['g']}
>>> from collections import Counter
>>> Counter(x) + Counter(y)
Counter({2: ['g'], 1: ['a', 'b', 'c', 'd', 'e', 'f']})
In Python 3.x, what if you run the same code, you will see:
Python 3.9.0 (v3.9.0:9cf6752276, Oct 5 2020, 11:29:23)
Type 'copyright', 'credits' or 'license' for more information
IPython 7.19.0 -- An enhanced Interactive Python. Type '?' for help.
In [1]: x={1:['a','b','c']}
In [2]: y={1:['d','e','f'],2:['g']}
In [3]: from collections import Counter
In [4]: Counter(x) + Counter(y)
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-4-0aa8b00837b3> in <module>
----> 1 Counter(x) + Counter(y)
/Library/Frameworks/Python.framework/Versions/3.9/lib/python3.9/collections/__init__.py in __add__(self, other)
759 for elem, count in self.items():
760 newcount = count + other[elem]
--> 761 if newcount > 0:
762 result[elem] = newcount
763 for elem, count in other.items():
TypeError: '>' not supported between instances of 'list' and 'int'
Let's take a look at the source code at vim /Library/Frameworks/Python.framework/Versions/3.9/lib/python3.9/collections/__init__.py +761
To check the different between Python2.x and Python3.x, we will find the path of collections in python2. to do that just simply execute a wrong call like:
>>> Counter(9,1) # wrong way to call.
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/collections.py", line 475, in __init__
raise TypeError('expected at most 1 arguments, got %d' % len(args))
TypeError: expected at most 1 arguments, got 2
and then, you see /System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/collections.py
.
Okay, now open both files and locate to the __add__
function in class Counter
.
vim -d /Library/Frameworks/Python.framework/Versions/3.9/lib/python3.9/collections/__init__.py +761 /System/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/collections.py
But we could find the deferent in this snipe code.
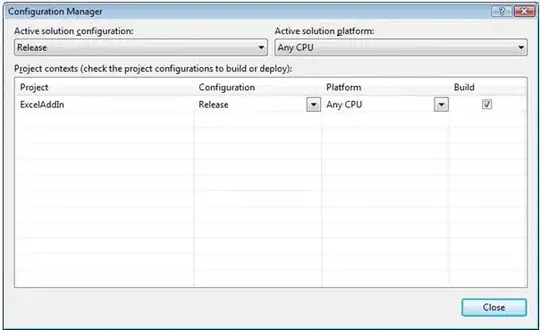
Think about this, you can see the TypeError: '>' not supported between instances of 'list' and 'int'
show two important things:
>
reflect to def __gt__(self, value, /)
method in class dict
. so let's check the different between python2 and python3 dict
.
'list'
and 'int'
. to make sure we meet the same type here, just add a line to print it out. before do compare line if newcount > 0:
like this way:
print("newcount is --> ", newcount, type(newcount))
if newcount > 0:
and you will see this:
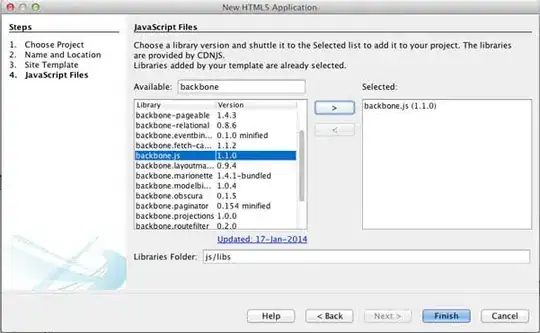
Not surprise, right? we could compare list
to int
, em makes sense..
let's quickly compare a list
with int
in python 2.
It's returning a True
. keep dived into the Python3.x
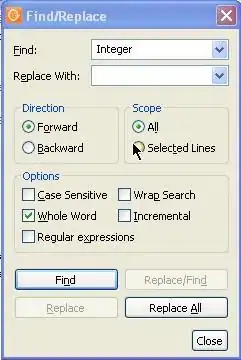
Okay, now you should be clear about what's going on. to fix this, should be easily do some change on your own python env or commit it to git.
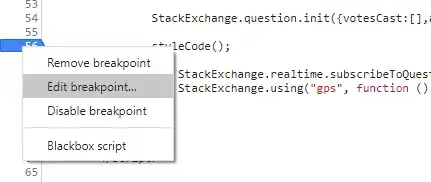
Finally, you got two good news, you fix the Python3.x bug. and you got your result.
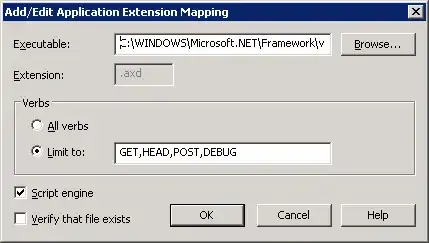
If desired result is a dict. You can use the following:
z = dict(Counter(x) + Counter(y))