Before starting with the answers to your questions, lets get it clear that arrays are "Objects" in Java.
So if you say int a[]= {1,2,3,4};
, you are creating an Object of type int
array, and to refer to that object, you will be using the reference a
. So now, lets to your questions :
1) What does it mean for a reference to refer to an array? An array is a number of elements while a reference is a single element. So what is the meaning of this reference?
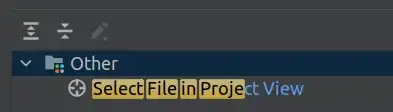
In the above image, a
is a reference, which is stored on the stack, whereas the actual array,i.e. the object to which a
refers, is stored on heap.
There is a class for every array type, so there's a class for int[]
. If you are curious, here's a code snippet that will prove my above statement :
public static void main(String[] args)
{
test(int[].class);
}
static void test(Class clazz)
{
System.out.println(clazz.getName());
System.out.println(clazz.getSuperclass());
for(Class face : clazz.getInterfaces())
System.out.println(face);
}
Credits for the above snippet.
Now, it is clear that JVM makes these classes itself at runtime.
And every class in java, is a subtype of the Object
class. Hence, a reference of type Object
can refer to the instance of type int[]
I hope this clears the first part of the question.
Now, the second part,
2) Is there a way to access the elements of the array using the reference?
Answer : Yes it is. The way is :
int c[] = (int[])oRef;
When you write the above statement, you create a reference of type int[]
, and you make that reference point to the previously created object of type int[]
. By saying (int[])oRef
, you are just typecasting the reference of type Object
which is a super class to int[]
which is the subclass.
So now the above picture will change to :
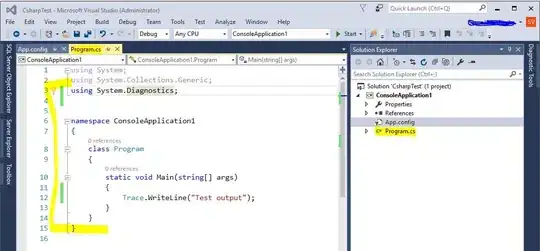
Hope that answers both your questions.