You could use the onchange
event bound to the form and save the data to a text file any time there is a change. This is done by silently submitting the form through an AJAX request to your server.
Javascript and PHP solution:
<form id="stackoverflow" method="POST" action="" onchange="save()">
<input name="subject" type="text" value="Hello World" style="width:222px"><br/>
<textarea name="message" style="width:220px;height:100px">This is an example of sending an AJAX request to PHP and writing it to file.</textarea><br/>
<button type="button" onclick='save()'>Submit</button>
</form><script>
function save() {
var form = document.querySelector('#stackoverflow');
var data = new FormData(form);
var req = new XMLHttpRequest();
req.open("POST", "form-write.php", true);
req.send(data);
console.log("..saved");
}
</script>
This method will send data to your server's PHP file every time someone changes something on one of your forms. A simple keypress will fire this event. The PHP file can do whatever you'd like, in this case we're just going to write the contents of your form fields to a simple text file.
PHP Code:
$subject = $_POST['subject'];
$message = $_POST['message'];
$output = "Subject: {$subject}\n";
$output.= "Message: {$message}";
file_put_contents("form.txt",$output);
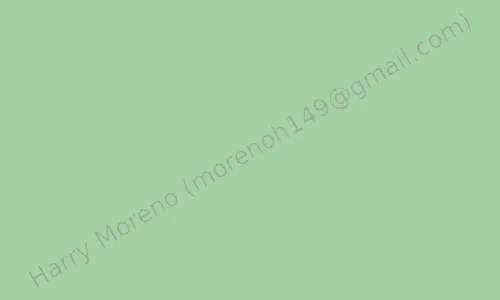
Example output:
Subject: Hello World
Message: This is an example of sending an AJAX request to PHP and writing it to file.
jQuery and PHP solution:
<script src="https://code.jquery.com/jquery-2.1.4.min.js"></script>
<form id="stackoverflow" method="POST" action="">
<input name="subject" type="text" value="Hello World" style="width:222px"><br/>
<textarea name="message" style="width:220px;height:100px">This is an example of sending an AJAX request to PHP and writing it to file.</textarea><br/>
<button type="button" onclick='save()'>Submit</button>
</form><script>
$("#stackoverflow").change(function(){
save();
});
function save() {
$.post("form-write.php", $('#stackoverflow').serialize() );
console.log("..saved");
}
</script>
Here we're going to store the contents of your form in JSON format because it is the easiest for us to work with. The JSON file will contain all of the fields in the form, and we can read it later with PHP using json_decode()
PHP Code:
file_put_contents("form.txt",json_encode($_POST));
An example of a JSON save would look like this:
{"subject":"Hello World","message":"This is an example of what JSON looks like."}
Read more about jQuery.post()