Why ?
Because char a[2][5]
is a 2 dimensional array stored as 10 contiguous chars. But parameter char **str
expects an array of pointers to one dimensional arrays of chars.
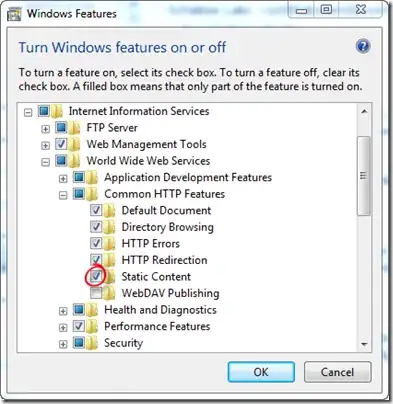
Solution 1:
Keep the function as it is, but pass it an array of pointers to char:
...
char *str1[2];
str1[0]=a[0];
str1[1]=a[1];
test(str1);
Solution 2:
CHange the function to accept 2D arrays with 5 elements in the secund dimension (to pass an n dimensional array the last n-1 dimensions must be predefined):
void test(char str[][5]) {
...
}
int main() {
...
test(a);
}
The inconvenience is that it is less flexible.
Solution 3
If your problem is specifically related to array of strings and not the more general issue of 2D array, switch from char*
to std::string
and you'll make your life easier !
void test(string str[]) {
...
}
int main(int argc, char* argv[]) {
string a[2] ={ "abc","def" };
test(a);
}