For changing text filed height declare NSLayoutConstraint variable constraint in your controller.
In .h file:
__weak IBOutlet NSLayoutConstraint *heightConstraintOfMyView;//height of the view
__weak IBOutlet NSLayoutConstraint *verticalspaceConstraintOfMyViewWithTopView;//space between myview and the view just above it
__weak IBOutlet NSLayoutConstraint *verticalSpaceConstraintOfMyViewWithBottomView;//space between myview and the view just below it
In your storyboard
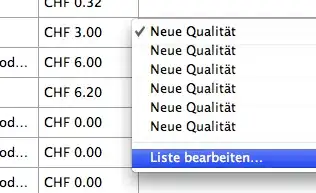
Connected
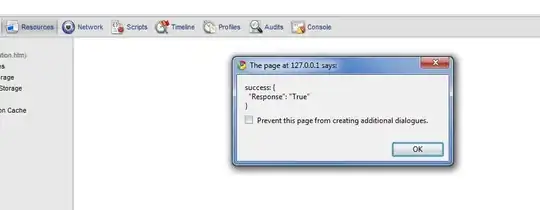
Vary the frame using constraints.
if (myConditionTrueForShowingView) {
//setting the constraint to update height
heightConstraintOfMyView = 40;
verticalspaceConstraintOfMyViewWithTopView.constant=20;
verticalSpaceConstraintOfMyViewWithBottomView.constant=80;
}
else{
//setting the constraint to update height
heightConstraintOfMyView = 0;
verticalspaceConstraintOfMyViewWithTopView.constant=20;
verticalSpaceConstraintOfMyViewWithBottomView.constant=0;
}
You can also register for keyboard notifications.
- (void)registerForKeyboardNotifications
{
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(keyboardWasShown:)
name:UIKeyboardDidShowNotification object:nil];
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(keyboardWillBeHidden:)
name:UIKeyboardWillHideNotification object:nil];
}
And Handle the height changes in the notifications call back-
@property (weak, nonatomic) IBOutlet NSLayoutConstraint *tableBottomSpaceConstrain;
-(void)keyboardWasShown:(NSNotification*)aNotification
{
self.view.translatesAutoresizingMaskIntoConstraints = YES;
if (self.tableBottomSpaceConstrain.constant) {//
return;
}
self.tableBottomSpaceConstrain.constant = 216;// Default Keyboard height is 216, varies for third party keyboard
[self.view setNeedsUpdateConstraints];
[self.view updateConstraints];
[self.view layoutIfNeeded];
[self.view setNeedsLayout];
[self.view setNeedsUpdateConstraints];
}
- (void)keyboardWillBeHidden:(NSNotification*)aNotification
{
self.tableBottomSpaceConstrain.constant =0;
[self.view layoutIfNeeded];
[self.view setNeedsLayout];
[self.view updateConstraints];
}
Learn more about NSLayoutConstraint
Implementing AutoLayout Constraints in Code
See Apple Doc
You can use some third party code if textfield is part of tableviewTPKeyboardAvoiding
See this link: How to make a UITextField move up when keyboard is present?
Making a UITableView scroll when text field is selected