There is a scroll view for iOS also in the interface builder object library:
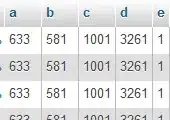
Start to finish here is how to make it work in storyboard.
1: go to you view controller and click on Attribute Inspector.
2: change Size to Freeform instead of Inferred.
3: Go to the main view on that storyboard, not your scrollview but
rather the top level view.
4: Click Size Inspector and set this view to your desired size. I
changed my height to 1000.
Now you will see that you storyboard has your view setup so you can
see the entire height of your scroll for easy design.
5: Drop on a scrollview and stretch it so it takes up the whole view.
You should now have a scrollview with size of 320,1000 sitting on a
view in your view controller.
Now we need to make it scroll and need to make it show content
correctly.
6: Click on your scrollview and click on Identity Inspector.
7: Add a User Defined runtime attribute with KeyPath of contentSize
then type of SIZE and put in your content size. For me it is (320,
1000).
Since we want to see our whole scroll view on the storyboard we
stretched it and it has a frame of 320,1000 but in order for this to
work in our app we need to change the frame down to what the visible
scrollview will be.
8: Add a runtime attribute with KeyPath frame with Type RECT and
0,0,320,416.
Now when we run our app we will have a visible scrollview has a frame
of 0,0,320, 416 and can scroll down to 1000. We are able to layout our
subviews and images and whatnot in Storyboard just the way we want
them to appear. Then our runtime attributes make sure to display it
properly. All of this without 1 line of code.
Is this what you were thinking of?
If you want the scroll view to change size I would recommend trying this:
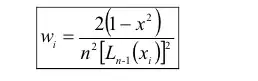
You want to do is drop the scroll view onto the view controller and and add constraints.
I have never used a scroll view before, so this might not work.