Looking at the bigger picture, of counting the dots on a dice, I would look at using ImageMagick - with the C++ binding called Magick++
from here
I would be looking at using "Blob Analysis", or "Connected Component Analysis" to count the dots on a dice.
Using this dice...
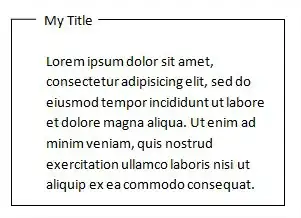
If I use ImageMagick at the command line like this:
convert dice.png -colorspace gray -threshold 50% \
-define connected-components:verbose=true \
-define connected-components:area-threshold=10 \
-connected-components 8 -auto-level output.png
Output
Objects (id: bounding-box centroid area mean-color):
0: 380x362+0+0 189.6,180.0 103867 srgba(255,255,255,1)
2: 93x92+248+32 293.8,77.5 6743 srgba(0,0,0,1)
4: 92x93+39+241 84.8,286.7 6741 srgba(0,0,0,1)
5: 93x93+248+241 293.8,286.8 6738 srgba(0,0,0,1)
1: 92x92+39+32 84.8,77.5 6736 srgba(0,0,0,1)
3: 93x93+143+136 189.3,182.1 6735 srgba(0,0,0,1)
You can see it has found 5 dots (the first one is actually the whole, white image), and I can put a red box around each dot like this so you can see what it has found:
convert dice.png -stroke red -fill none -strokewidth 1 -draw "rectangle 248,32 341,124" -draw "rectangle 39,241 131,334" -draw "rectangle 248,241 341,334" -draw "rectangle 39,32 131,124" -draw "rectangle 143,136 236,229" result.png
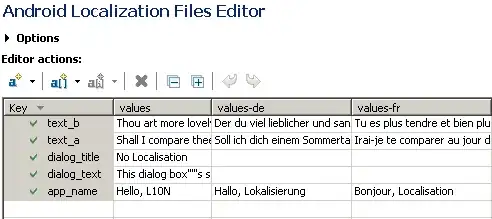