Take your data points and create a Math.NET Numerics Cubic Spline object. Then use the .Differentiate()
method to get the slope at each point you want.
Example
Try the code below:
static class Program
{
const int column_width = 12;
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main(string[] args)
{
var xvec = new DenseVector(new double[] { 0.0, 1.0, 2.0, 3.0, 4.0 });
var yvec = new DenseVector(new double[] { 3.0, 2.7, 2.3, 1.6, 0.2 });
Debug.WriteLine("Input Data Table");
Debug.WriteLine($"{"x",column_width} {"y",column_width}");
for(int i = 0; i < xvec.Count; i++)
{
Debug.WriteLine($"{xvec[i],column_width:G5} {yvec[i],column_width:G5}");
}
Debug.WriteLine(" ");
var cs = CubicSpline.InterpolateNatural(xvec, yvec);
var x = new DenseVector(15);
var y = new DenseVector(x.Count);
var dydx = new DenseVector(x.Count);
Debug.WriteLine("Interpoaltion Results Table");
Debug.WriteLine($"{"x",column_width} {"y",column_width} {"dy/dx",column_width}");
for(int i = 0; i < x.Count; i++)
{
x[i] = (4.0*i)/(x.Count-1);
y[i] = cs.Interpolate(x[i]);
dydx[i] = cs.Differentiate(x[i]);
Debug.WriteLine($"{x[i],column_width:G5} {y[i],column_width:G5} {dydx[i],column_width:G5}");
}
}
}
And look at the debug output:
Input Data Table
x y
0 3
1 2.7
2 2.3
3 1.6
4 0.2
Interpoaltion Results Table
x y dy/dx
0 3 -0.28214
0.28571 2.919 -0.28652
0.57143 2.8354 -0.29964
0.85714 2.7469 -0.3215
1.1429 2.6509 -0.35168
1.4286 2.5454 -0.38754
1.7143 2.429 -0.42864
2 2.3 -0.475
2.2857 2.154 -0.55809
2.5714 1.9746 -0.7094
2.8571 1.7422 -0.92894
3.1429 1.4382 -1.1979
3.4286 1.0646 -1.4034
3.7143 0.64404 -1.5267
4 0.2 -1.5679
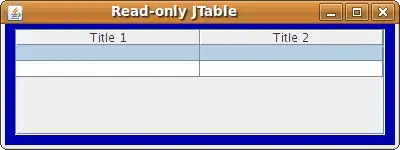