You are making a number of mistakes.
Firstly, less of a mistake, but matplotlib.pylab
is supposedly used to access matplotlib.pyplot
and numpy
together (for a more matlab-like experience), I think it's more suggested to use matplotlib.pyplot as plt
in scripts (see also this Q&A).
Secondly, your angles are in degrees, but math functions by default expect radians. You have to convert your angles to radians before passing them to the trigonometric functions.
Thirdly, your current code sets t1
to have a single time point for every angle. This is not what you need: you need to compute the maximum time t
for every angle (which you did in t
), then for each angle create a time vector from 0
to t
for plotting!
Lastly, you need to use the same plotting time vector in both terms of y
, since that's the solution to your mechanics problem:
y(t) = v_{0y}*t - g/2*t^2
This assumes that g
is positive, which is again wrong in your code. Unless you set the y
axis to point downwards, but the word "projectile" makes me think this is not the case.
So here's what I'd do:
import numpy as np
import matplotlib.pyplot as plt
#initialize variables
#velocity, gravity
v = 30
g = 9.81 #improved g to standard precision, set it to positive
#increment theta 25 to 60 then find t, x, y
#define x and y as arrays
theta = np.arange(25,65,5)[None,:]/180.0*np.pi #convert to radians, watch out for modulo division
plt.figure()
tmax = ((2 * v) * np.sin(theta)) / g
timemat = tmax*np.linspace(0,1,100)[:,None] #create time vectors for each angle
x = ((v * timemat) * np.cos(theta))
y = ((v * timemat) * np.sin(theta)) - ((0.5 * g) * (timemat ** 2))
plt.plot(x,y) #plot each dataset: columns of x and columns of y
plt.ylim([0,35])
plot.show()
I made use of the fact that plt.plot
will plot the columns of two matrix inputs versus each other, so no loop over angles is necessary. I also used [None,:]
and [:,None]
to turn 1d numpy array
s to 2d row and column vectors, respectively. By multiplying a row vector and a column vector, array broadcasting ensures that the resulting matrix behaves the way we want it (i.e. each column of timemat
goes from 0
to the corresponding tmax
in 100 steps)
Result:
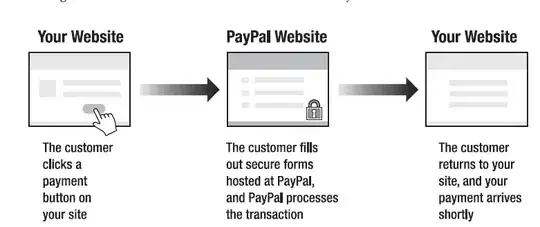